Facebook's FBConnect SDK issues on iOS
Solution 1
First:
[facebook authorize:[NSArray arrayWithObjects:@"publish_stream",@"offline_access",nil] delegate:self];
The offline_access
key here will keep your auth token alive forever (or, more specifically, until the user manually de-authorizes your application in their application settings in their Facebook account settings). Also, set your active VC as the delegate (more on that later).
Secondly:
-(void)popUserShareFeed {
NSMutableDictionary *dictionary = [NSMutableDictionary dictionary];
//Giving the dictionary some parameters for posting defaults
[facebook dialog:@"feed" andParams:dictionary andDelegate:self];
}
Call this method (or one like it) in your -fbDidLogin
delegate method. Also call it in your original method if the session was still valid, i.e.:
if (![facebook isSessionValid]) {
[facebook authorize:[NSArray arrayWithObjects:@"publish_stream", nil] delegate:(MyAppDelegate *)[[UIApplication sharedApplication] delegate]];
} else {
[self popUserShareFeed];
}
...and your new fbDidLogin
:
- (void)fbDidLogin
{
NSUserDefaults *defaults = [NSUserDefaults standardUserDefaults];
[defaults setObject:[facebook accessToken] forKey:@"FBAccessTokenKey"];
[defaults setObject:[facebook expirationDate] forKey:@"FBExpirationDateKey"];
[defaults synchronize];
[self popUserShareFeed];
}
(Note: you'll have to define this in MyViewController.m
and use it as your FBSessionDelegate
. It will be functionally equivalent. Your AppDelegate
does not need to also be your FBSessionDelegate
.
Third:
Implement the -fbDidNotLogin:(BOOL)cancelled
FBSessionDelegate
method, like so:
-(void)fbDidNotLogin:(BOOL)cancelled {
if (cancelled) {
... some alert about the user cancelling...
} else {
... some alert about how it failed for some reason...
}
}
Fourth, as far as your bizarro errors go: A) the Facebook SDKs in general are not great, and B) I'd only set the auth token and the expiration date on your facebook
object if
A) you don't already have one instantiated (i.e., it's nil
)
and
B) the expirationDate you're setting is in the future (i.e. timeIntervalSinceNow
[the NSDate
instance method] called on it returns > 0).
Solution 2
Sounds like you're experiencing the same issue described in this Facebook Platform Developer forum post. I'm encountering the same problem, but on the web. Only one response was given from Facebook in that thread, and it's wrong information.
Facebook has the worst developer docs and developer support ever, I wouldn't hold my breath waiting on a solution.
Solution 3
I had similar but not the same problem: message "An error occurred. Please try again later." was shown always.
The problem was with not properly configured App ID - it was provided by customer (so I'm not sure what exactly was wrong); everything works properly since I replaced it with my own test App ID (from previous app).
Related videos on Youtube
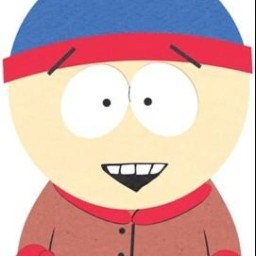
Niv
Updated on June 04, 2022Comments
-
Niv 7 months
I'm using FBConnect sdk in order to publish posts to a user's profile via my application. I'm having a number of problems with this:
When the relevant code runs for the first time on the device, the user is re-directed, as wanted, to the facebook app/website, which asks him to authorize it. if the user authorizes it, it returns back to the application, which pops a "Connect to facebook" view controller which asks the user to log in. this is weird, as the user is already logged in, otherwise how could he authorize the app? but I guess this may be ok, as he hadn't logged in through the app yet. after he logs in, it does nothing. only the second time the code gets run, after he authorized the app, the user gets the posting dialog.
If the user hadn't authorized the app, when it comes back to my app after the authorization dialog, it asks the user to login ( just as if he authorized ), and does nothing after he had logged in. only the second time the code gets ran, the authorization dialog opens, with the optinos "Authorize" & "Leave App", instead of "Authorize" & "Don't authorize" / "Allow" & "Don't Allow".In addition, if the user has deleted his authorization via his account's settings on facebook, instead of just asking him to re-authorize it, a facebook dialog pops ( instead of the post/authorization dialog ), saying: "An error occurred. Please try again later." Trying later doesn't help. it will pop always, even if u restart the app. the only way to make it go away is to re-install the app, which will cause it to re-pop the authoriziation dialog.
So here's what I want to achieve:- After the user authorizes the app, he wouldn't have to log in again.
- After the user authorizes the app, the posting dialog will pop immedietly, without him having to re-run the code ( which is triggered, btw, with a button ).
- If the user un-authorizes the app, he will be prompted again with the authorization dialog, instead of the error dialog
- If he refused the authorization, I will call a function that displays an error/etc.
Here's the relevant code:
MyViewController.m
- (void)shareOnFacebook { Facebook *facebook = [[Facebook alloc] initWithAppId:myAppID]; [(MyAppDelegate *)[[UIApplication sharedApplication] delegate] setFacebook:facebook]; [facebook release]; facebook = [(MyAppDelegate *)[[UIApplication sharedApplication] delegate] facebook]; NSUserDefaults *defaults = [NSUserDefaults standardUserDefaults]; if ([defaults objectForKey:@"FBAccessTokenKey"] && [defaults objectForKey:@"FBExpirationDateKey"]) { facebook.accessToken = [defaults objectForKey:@"FBAccessTokenKey"]; facebook.expirationDate = [defaults objectForKey:@"FBExpirationDateKey"]; } if (![facebook isSessionValid]) { [facebook authorize:[NSArray arrayWithObjects:@"publish_stream", nil] delegate:(MyAppDelegate *)[[UIApplication sharedApplication] delegate]]; } NSMutableDictionary *dictionary = [NSMutableDictionary dictionary]; //Giving the dictionary some parameters for posting defaults [facebook dialog:@"feed" andParams:dictionary andDelegate:self]; //Note: we have 2 different delegates! appDelegate for connections & url switching, and self for dialogs }
MyAppDelegate.h
@interface MyAppDelegate : NSObject <UIApplicationDelegate, FBSessionDelegate, FBDialogDelegate> { Facebook *facebook; // kept for facebook sharing, accessed only from MyViewController although delegate methods are handeled in here } @property (nonatomic, retain) Facebook *facebook; @end
MyAppDelegate.m
- (void)fbDidLogin { NSUserDefaults *defaults = [NSUserDefaults standardUserDefaults]; [defaults setObject:[facebook accessToken] forKey:@"FBAccessTokenKey"]; [defaults setObject:[facebook expirationDate] forKey:@"FBExpirationDateKey"]; [defaults synchronize]; } - (BOOL)application:(UIApplication *)application handleOpenURL:(NSURL *)url { return [self.facebook handleOpenURL:url]; }
Is it possible that I'm missing a couple of delegate functions on MyViewController? Because I see for some reason I've marked it as implementing the FBDialogDelegate protocol, although he doesn't implement any function from there.
I'd be really glad if you guys would help me, as this is extremely frustrating for me. I couldn't find nothing about this on the internet, and I feel like im drowning in here.
Tnx in advance! - After the user authorizes the app, he wouldn't have to log in again.
-
Niv over 11 yearscan you provide more code/information? I couldn't find this class. All I got is Facebook, FBDialog, FBLoginDialog and FBRequest.
-
Avi Shukron over 11 years@Niv He's using the old facebook SDK - FBConnect. You are using the new one - Facebook iOS SDK.
-
Adam about 11 yearsI'm getting this error from ShareKit itself (this page was one of the first hits I got on Google, trying to debug ShareKit). ShareKit is terrible - the sum total of the docs for Facebook say "there is no setup for Facebook", which is patently untrue. I would recommend avoiding it - I'm about to switch back to the FB iOS SDK because ShareKit is so appallingly bad.