FacebookSDK for Unity iOS Mach-O linker error - undefined symbols _iosLogin,
Solution 1
Those symbols are exported from FacebookSDK.a, which should be getting included into your Xcode project by the [PostProcessBuild] step in /Assets/Facebook/Editor/FacebookPostprocess.cs. That file actually spins through all of the files in your project that end with ".projmods" in their filename and applies them as transformations to your xcode project. I've seen 2 things go wrong here when having this kind of problem:
- I had removed either FacebookPostprocess.cs or some of the ".projmods" files from my project accidentally. Check that both exist. The specific projmods file in this case should be at /Assets/Facebook/Editor/iOS.
- You have your own [PostProcessBuild] steps that clobber Facebook's changes. You can fix this by applying the optional "order" parameter to the PostProcessBuild attribute.
You can also check in Xcode that FacebookSDK.a is, indeed, in the /Libraries group of the project and that under "Build Phases" it is included as a binary to link with.
Also look carefully at the log output from Unity when you build. Sometimes the projmods postprocessor will complain about files not found, etc.
Solution 2
One other potential cause of this: the Facebook SDK uses a third-party library XCode-Editor-for-Unity to do it's XCode project postprocessing. If any other middleware in your project uses this as well, you'll potentially have duplicate instances of it.
In our case, the SponsorPay api also had a copy of this library, and when importing the Facebook API it was overwriting SponsorPay's implementation of it (which had some modifications from the original library).
This took a few steps to fix:
First, move each instance to its own namespace, and fix the references to them:
in Facebook/Editor/iOS/XCodeEditor-for-Unity/*.cs:
namespace UnityEditor.XCodeEditor.Facebook
{
...
}
and in Facebook/Editor/FacebookPostprocess.cs:
// our 'XCProject' is now in the UnityEditor.XCodeEditor.Facebook namespace
UnityEditor.XCodeEditor.Facebook.XCProject project =
new UnityEditor.XCodeEditor.Facebook.XCProject(path);
... And do the same for the other library.
Once we had this fixed, we ran into one last problem: Each library's Postprocess script was walking over the entire project to find .projmods
files, which meant each of these files got processed twice (once by the wrong implementation, which triggered another crash).
So, one last step, make sure each of the libraries is postprocessing only its own path:
// Find and run through all projmods files to patch the project
// Set this to your project's Facebook API location:
string sdkPath = "Facebook/Editor/iOS";
string projModPath = System.IO.Path.Combine(Application.dataPath, sdkPath)
var files = System.IO.Directory.GetFiles(projModPath, "*.projmods", System.IO.SearchOption.AllDirectories);
foreach (var file in files)
{
project.ApplyMod(Application.dataPath, file);
}
project.Save();
and again, doublecheck this for the other library as well.
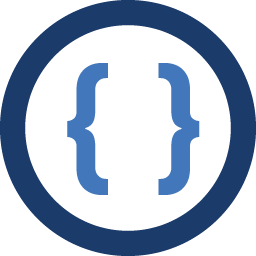
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I've added FacebookSDK into my Unity project according to tutorial here https://developers.facebook.com/docs/unity/getting-started/canvas/.
It means that I've downloaded the SDK .unitypackage, imported it into Unity project and set application name and ID in configuration settings inspector.
When I try to build for device, Xcode build hangs on following Mach-O linker error:
Undefined symbols for architecture armv7: "_iosLogin", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosLogout", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosInit", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosSetShareDialogMode", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosFeedRequest", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosAppRequest", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosFBSettingsPublishInstall", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosFBAppEventsSetLimitEventUsage", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosGetDeepLink", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosFBAppEventsLogPurchase", referenced from: RegisterMonoModules() in RegisterMonoModules.o "_iosFBAppEventsLogEvent", referenced from: RegisterMonoModules() in RegisterMonoModules.o ld: symbol(s) not found for architecture armv7 clang: error: linker command failed with exit code 1 (use -v to see invocation)
It looks like Unity, Xcode or me forgot to include some library, search path or source file somewhere. Can you help me to find out what happened wrong? Do you know in which file this symbols should be or how to setup linker?
Unity 4.3.0f4 (also tried 4.2.?) Facebook SDK 4.3.4 (also tried 4.3.3) Xcode 5.0.2, deployment target 4.0 (also tried 6.1)
-
Admin over 10 yearsThank you for your advices. I've checked the Unity log as you suggested and found an error in Facebook/Editor/iOS/third_party/XCodeEditor-for-Unity/PBXParser.cs in ParseDictionary method. There was an attemp to dictionary.Add() with duplicate key on line 184. I think there is problem with some configuration not file itself but I have added check for duplicate keys, and then I am able to build. But just for the first time (or after deleting iOS build folder). Not to build over existing project. If I try to second(third,...) build&run, Unity generates malformed xml Info.plist.
-
Admin over 10 yearsOne another finding: when I build for second time with Replace instead of Append it builds without error, so it is probably another error. So I consider it resolved. Maybe it could be usable if you have any idea why ParseDictionary tries to add duplicate keys or where does it get data from but it is not necessary.