failed to lazily initialize a collection of role: User.authorities, could not initialize proxy - no Session
10,028
Solution 1
Give it a try;
In Service;
User user = userService.findByUsername(username);
user.getAuthorities().size(); //You may need call this line in userService.findByUsername(username); method
In User;
public Set<Authority> getAuthorities(){
return authorities;
}
Solution 2
just initialize authority manually as below:
User user = userService.findByUsername(username).orElseThrow(() -> new UsernameNotFoundException("Username not found"));
Set<Authority> authorities = user.getAuthorities();
Hibernate.initialize(authorities);
return user;
Related videos on Youtube
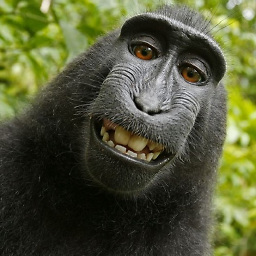
Author by
alexanoid
Updated on September 16, 2022Comments
-
alexanoid 9 months
In my Spring Boot/Data/JPA application I have a following entity:
@Entity @NamedEntityGraph(name = "graph.User", attributeNodes = { @NamedAttributeNode("authorities") }) @Table(name = "users") public class User extends BaseEntity implements UserDetails { private static final long serialVersionUID = 8884184875433252086L; @Id @SequenceGenerator(name = "users_id_seq", sequenceName = "users_id_seq", allocationSize = 1) @GeneratedValue(strategy = GenerationType.AUTO, generator = "users_id_seq") private Long id; ... @JsonIgnore @ManyToMany(fetch = FetchType.LAZY) @JoinTable(name = "users_authorities", joinColumns = { @JoinColumn(name = "user_id") }, inverseJoinColumns = { @JoinColumn(name = "authority_id") }) private Set<Authority> authorities = new HashSet<Authority>(); }
This is my Spring
AuthenticationService
:@Service public class AuthenticationService implements UserDetailsService { @Autowired private UserService userService; @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { User user = userService.findByUsername(username); if (user == null) { throw new UsernameNotFoundException(String.format("User %s does not exist!", username)); } return user; } }
During the application startup I'm receiving following exception:
org.hibernate.LazyInitializationException: failed to lazily initialize a collection of role: com.example.common.model.user.User.authorities, could not initialize proxy - no Session at org.hibernate.collection.internal.AbstractPersistentCollection.throwLazyInitializationException(AbstractPersistentCollection.java:576) at org.hibernate.collection.internal.AbstractPersistentCollection.withTemporarySessionIfNeeded(AbstractPersistentCollection.java:215) at org.hibernate.collection.internal.AbstractPersistentCollection.initialize(AbstractPersistentCollection.java:555) at org.hibernate.collection.internal.AbstractPersistentCollection.read(AbstractPersistentCollection.java:143) at org.hibernate.collection.internal.PersistentSet.iterator(PersistentSet.java:180) at org.springframework.security.authentication.AbstractAuthenticationToken.<init>(AbstractAuthenticationToken.java:61) at org.springframework.security.authentication.UsernamePasswordAuthenticationToken.<init>(UsernamePasswordAuthenticationToken.java:72) at org.springframework.security.authentication.dao.AbstractUserDetailsAuthenticationProvider.createSuccessAuthentication(AbstractUserDetailsAuthenticationProvider.java:224) at org.springframework.security.authentication.dao.AbstractUserDetailsAuthenticationProvider.authenticate(AbstractUserDetailsAuthenticationProvider.java:196) at org.springframework.security.authentication.ProviderManager.authenticate(ProviderManager.java:167) at org.springframework.security.authentication.ProviderManager.authenticate(ProviderManager.java:192) at org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter.attemptAuthentication(UsernamePasswordAuthenticationFilter.java:93) at org.springframework.security.web.authentication.AbstractAuthenticationProcessingFilter.doFilter(AbstractAuthenticationProcessingFilter.java:217) at org.springframework.security.web.FilterChainProxy$VirtualFilterChain.doFilter(FilterChainProxy.java:330) at org.springframework.security.web.authentication.logout.LogoutFilter.doFilter(LogoutFilter.java:120) at org.springframework.security.web.FilterChainProxy$VirtualFilterChain.doFilter(FilterChainProxy.java:330) at org.springframework.security.web.header.HeaderWriterFilter.doFilterInternal(HeaderWriterFilter.java:64) at org.springframework.web.filter.OncePerRequestFilter.doFilter(OncePerRequestFilter.java:107) at org.springframework.security.web.FilterChainProxy$VirtualFilterChain.doFilter(FilterChainProxy.java:330) at org.springframework.security.web.context.SecurityContextPersistenceFilter.doFilter(SecurityContextPersistenceFilter.java:91) at org.springframework.security.web.FilterChainProxy$VirtualFilterChain.doFilter(FilterChainProxy.java:330) at org.springframework.security.web.context.request.async.WebAsyncManagerIntegrationFilter.doFilterInternal(WebAsyncManagerIntegrationFilter.java:53) at org.springframework.web.filter.OncePerRequestFilter.doFilter(OncePerRequestFilter.java:107) at org.springframework.security.web.FilterChainProxy$VirtualFilterChain.doFilter(FilterChainProxy.java:330) at org.springframework.security.web.FilterChainProxy.doFilterInternal(FilterChainProxy.java:213) at org.springframework.security.web.FilterChainProxy.doFilter(FilterChainProxy.java:176) at org.springframework.web.filter.DelegatingFilterProxy.invokeDelegate(DelegatingFilterProxy.java:346) at org.springframework.web.filter.DelegatingFilterProxy.doFilter(DelegatingFilterProxy.java:262) at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:240) at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:207) at org.springframework.web.filter.CharacterEncodingFilter.doFilterInternal(CharacterEncodingFilter.java:121) at org.springframework.web.filter.OncePerRequestFilter.doFilter(OncePerRequestFilter.java:107) at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:240) at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:207) at org.springframework.boot.actuate.autoconfigure.MetricsFilter.doFilterInternal(MetricsFilter.java:103) at org.springframework.web.filter.OncePerRequestFilter.doFilter(OncePerRequestFilter.java:107) at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:240) at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:207) at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:212) at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:106) at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:502) at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:141) at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:79) at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:88) at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:522) at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:1095) at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:672) at org.apache.tomcat.util.net.NioEndpoint$SocketProcessor.doRun(NioEndpoint.java:1500) at org.apache.tomcat.util.net.NioEndpoint$SocketProcessor.run(NioEndpoint.java:1456) at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1142) at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:617) at org.apache.tomcat.util.threads.TaskThread$WrappingRunnable.run(TaskThread.java:61) at java.lang.Thread.run(Thread.java:745)
How to configure my application in order to correctly handle
User.authorities
withFetchType.LAZY
? -
alexanoid about 7 yearsUnfortunately the same result - failed to lazily initialize a collection of role
-
Yusuf K. about 7 years@alexanoid Did you try it in UserService instead AuthenticationService? and do you have transaction?
-
alexanoid about 7 yearsSorry, my bad! In UserService it is working fine! Is it a good place to go with ?
-
Yusuf K. about 7 yearsYes, We have to make hibernate get collections in session context.
-
fascynacja over 5 yearsthis solution works also for me, but is there any more elegant way to achieve that?
-
Yusuf K. over 5 years@fascynacja I think this is the best way. (better than using eager which is the other solution)
-
Nikolai Shevchenko over 2 yearsWhile it's a working solution, eager fetching is not always desired behavior