Fetch Candlestick/Kline data from Binance API using Python (preferably requests) to get JSON Dat
Solution 1
you are missing the mandatory parameters symbol and interval, the query should be like this:
https://api.binance.com/api/v3/klines?symbol=BTCUSDT&interval=1h
you need to import only requests:
import requests
market = 'BTCUSDT'
tick_interval = '1h'
url = 'https://api.binance.com/api/v3/klines?symbol='+market+'&interval='+tick_interval
data = requests.get(url).json()
print(data)
Please check the official Binance REST API documentation here: https://github.com/binance/binance-spot-api-docs/blob/master/rest-api.md
Solution 2
The requests python package has a params
, json
argument, so you don't need to import any of those packages you are importing.
import requests
url = 'https://api.binance.com/api/v3/klines'
params = {
'symbol': 'BTCUSDT',
'interval': '1h'
}
response = requests.get(url, params=params)
print(response.json())
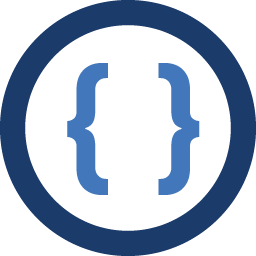
Admin
Updated on June 11, 2021Comments
-
Admin almost 3 years
I am developing a telegram bot that fetches Candlestick Data from Binance API. I am unable to get JSON Data as a response. The following code is something that I tried.
import requests import json import urllib.request `url = "https://api.binance.com/api/v1/klines" response = requests.request("GET", url) print(response.text)`
Desired Output:
[ [ 1499040000000, // Open time "0.01634790", // Open "0.80000000", // High "0.01575800", // Low "0.01577100", // Close "148976.11427815", // Volume 1499644799999, // Close time "2434.19055334", // Quote asset volume 308, // Number of trades "1756.87402397", // Taker buy base asset volume "28.46694368", // Taker buy quote asset volume "17928899.62484339" // Ignore ] ]
Question Edited:
The output that I am getting is:
`{"code":-1102,"msg":"Mandatory parameter 'symbol' was not sent, was empty/null, or malformed."}'
-
H Aßdøµ about 3 yearsIs it possible to pass a list of symbols?
-
Domenico about 3 years@HAßdøµ No you can't pass a list of symbols, please refer to the API documentation: github.com/binance/binance-spot-api-docs/blob/master/….
-
Domenico about 3 yearsthe last line should be: print(response.json()) instead of: print(response.json)