Fibonacci sequence in assembly language
Solution 1
I believe you just want to know how to create a loop in assembly. See the below for some examples:
x86 assembly programming loops with ecx and loop instruction versus jmp + j<condition>
Solution 2
.586
.MODEL FLAT
INCLUDE io.h ; header file for input/output
.STACK 4096
.DATA
resultLbl BYTE "The result is", 0
sum BYTE 11 DUP (?), 0
.CODE
_MainProc PROC
mov eax,1
mov ebx,1 ; will store answer
dtoa sum , eax
output resultLbl , sum
dtoa sum ,ebx
output resultLbl ,sum
mov ecx ,5
_for:
mov edx , eax
add edx , ebx
dtoa sum ,edx
output resultLbl ,sum
mov eax ,ebx
mov ebx ,edx
dec ecx
cmp ecx , 0
jne _for
mov eax , 0
ret
_MainProc ENDP
END
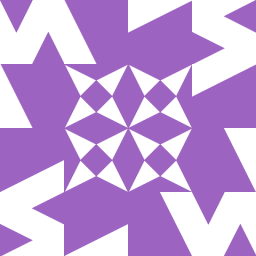
Shawn
Updated on November 24, 2022Comments
-
Shawn 29 days
Need some help understanding this more or less. We are just getting into loops and OFFSET operands and would appreciate some help. So here are my instructions:
Write an assembly language program using the LOOP instruction with indirect addressing mode that calculating the first 12 values in the Fibonacci number sequence, {1, 1, 2, 3, 5, 8, 13, …}. Place each value in the EAX register and display it with a call DumpRegs statement inside the loop.
Please use the following variable definition: Fibonacci BYTE 1, 1, 10 DUP (?)
Insert the following statements immediately after the loop. They will display the hexadecimal contents of the target string: mov esi, OFFSET Fibonacci ; offset the variables mov ebx,1 ; byte format mov ecx, SIZEOF Fibonacci ; counter call dumpMem ; display the data in the memory
If your program works correctly, you will see the following sequence of hexadecimal bytes on the screen when the program runs: 01 01 02 03 05 08 0D 15 22 37 59 90
.data Fibonacci BYTE 1, 1, 10 DUP (?) .code main PROC L1: mov esi, OFFSET Fibonacci ; offset the variables mov ebx,1 ; byte format mov ecx, SIZEOF Fibonacci ; counter call dumpMem ; display the data in the memory exit ;exits to Operating System main ENDP END main
So I know I need to make a loop statement, but am completely stuck on how to begin it. Any advice will be greatly appreciated!
-
Shawn over 9 yearsOk so I'm confused. I have this:
-
Shawn over 9 years.data Fibonacci BYTE 1, 1, 10 DUP (?) .code main PROC mov eax, 12 ;sets the loop count to 12 L1: mov count, eax ;saves the loop count Loop L1 ;repeat the loop in L1 mov esi, OFFSET Fibonacci ; offset the variables mov ebx,1 ; byte format mov ecx, SIZEOF Fibonacci ; counter call dumpMem ; display the data in the memory exit ;exits to Operating System main ENDP END main
-
ext about 5 yearsIt would be useful in you in addition to the code posted an explanation and possible some reference.