File upload using java websocket API and Javascript
Solution 1
After some researches and tries, I found out that 'reader.readAsBinaryString(file);' was a cause of Question 1. Changing it into 'reader.readAsArrayBuffer(file);' my first problem has been solved.
In addition, since websocket transfers a file as several partial data automatically, I changed source as below. This works! only when the file size is not so big. :/
Changed server side source:
package websocket;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
import javax.websocket.CloseReason;
import javax.websocket.EndpointConfig;
import javax.websocket.OnClose;
import javax.websocket.OnError;
import javax.websocket.OnMessage;
import javax.websocket.OnOpen;
import javax.websocket.Session;
import javax.websocket.server.ServerEndpoint;
@ServerEndpoint("/receive/fileserver")
public class FileServer {
static File uploadedFile = null;
static String fileName = null;
static FileOutputStream fos = null;
final static String filePath="d:/download/";
@OnOpen
public void open(Session session, EndpointConfig conf) {
System.out.println("chat ws server open");
}
@OnMessage
public void processUpload(ByteBuffer msg, boolean last, Session session) {
System.out.println("Binary Data");
while(msg.hasRemaining()) {
try {
fos.write(msg.get());
} catch (IOException e) {
e.printStackTrace();
}
}
}
@OnMessage
public void message(Session session, String msg) {
System.out.println("got msg: " + msg);
if(!msg.equals("end")) {
fileName=msg.substring(msg.indexOf(':')+1);
uploadedFile = new File(filePath+fileName);
try {
fos = new FileOutputStream(uploadedFile);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}else {
try {
fos.flush();
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@OnClose
public void close(Session session, CloseReason reason) {
System.out.println("socket closed: "+ reason.getReasonPhrase());
}
@OnError
public void error(Session session, Throwable t) {
t.printStackTrace();
}
}
Browser(Client) side:
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Chat</title>
<script type="text/javascript" src="/MyHomePage/jquery-2.0.3.min.js"></script>
</head>
<body>
<h2>File Upload</h2>
Select file
<input type="file" id="filename" />
<br>
<input type="button" value="Connect" onclick="connectChatServer()" />
<br>
<input type="button" value="Upload" onclick="sendFile()" />
<script>
var ws;
function connectChatServer() {
ws = new WebSocket(
"ws://localhost:8080/MyHomePage/receive/fileserver");
ws.binaryType = "arraybuffer";
ws.onopen = function() {
alert("Connected.")
};
ws.onmessage = function(evt) {
alert(evt.msg);
};
ws.onclose = function() {
alert("Connection is closed...");
};
ws.onerror = function(e) {
alert(e.msg);
}
}
function sendFile() {
var file = document.getElementById('filename').files[0];
ws.send('filename:'+file.name);
var reader = new FileReader();
var rawData = new ArrayBuffer();
//alert(file.name);
reader.loadend = function() {
}
reader.onload = function(e) {
rawData = e.target.result;
ws.send(rawData);
alert("the File has been transferred.")
ws.send('end');
}
reader.readAsArrayBuffer(file);
}
</script>
</body>
</html>
Still I cannot figure out how to transfer larger size file. (I'm suspecting auto-timeout and/or buffer size). Any advice plz?
Solution 2
In My case its working to upload large files without any browser crash or socket close.
Please follow the following steps to work it.
- Use web workers to slice the large files
- send the chunks to server using Websocket from main thread
- construct file on server side based on blob or chunk information.
- maintain unique id for each file while uploading files to server so that server can recognize to which file append
- In server side initially place it in temp folder with unique id after completion move it to the exact folder.
You can through this article to get an idea about the complete work flow, its working with Websocket.
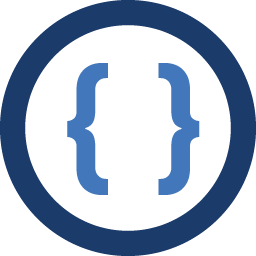
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm studying websocket and have done chat program with websocket/json. But I'm stuck at file uploading ATM. Any advice & answer would be thankful.
Server side:
package websocket; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.nio.ByteBuffer; import javax.websocket.CloseReason; import javax.websocket.EndpointConfig; import javax.websocket.OnClose; import javax.websocket.OnError; import javax.websocket.OnMessage; import javax.websocket.OnOpen; import javax.websocket.Session; import javax.websocket.server.ServerEndpoint; @ServerEndpoint("/receive/fileserver") public class FileServer { @OnOpen public void open(Session session, EndpointConfig conf) { System.out.println("chat ws server open"); } @OnMessage public void processUpload(ByteBuffer msg, boolean last, Session session) { System.out.println("Binary message"); FileOutputStream fos = null; File file = new File("D:/download/tmp.txt"); try { fos = new FileOutputStream(file); } catch (FileNotFoundException e) { e.printStackTrace(); } byte readdata = (byte) -999; while(readdata!=-1) { readdata=msg.get(); try { fos.write(readdata); } catch (IOException e) { e.printStackTrace(); } } } @OnMessage public void message(Session session, String msg) { System.out.println("got msg: " + msg + msg.length()); } @OnClose public void close(Session session, CloseReason reason) { System.out.println("socket closed: "+ reason.getReasonPhrase()); } @OnError public void error(Session session, Throwable t) { t.printStackTrace(); } }
Client:
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Chat</title> <script type="text/javascript" src="/MyHomePage/jquery-2.0.3.min.js"></script> </head> <body> <h2>File Upload</h2> Select file <input type="file" id="filename" /> <br> <input type="button" value="Connect" onclick="connectChatServer()" /> <br> <input type="button" value="Upload" onclick="sendFile()" /> <script> var ws; function connectChatServer() { ws = new WebSocket( "ws://localhost:8080/MyHomePage/receive/fileserver"); ws.binaryType = "arraybuffer"; ws.onopen = function() { alert("Connected.") }; ws.onmessage = function(evt) { alert(evt.msg); }; ws.onclose = function() { alert("Connection is closed..."); }; ws.onerror = function(e) { alert(e.msg); } } function sendFile() { var file = document.getElementById('filename').files[0]; var reader = new FileReader(); var rawData = new ArrayBuffer(); reader.loadend = function() { } reader.onload = function(e) { rawData = e.target.result; ws.send(rawData); alert("the File has been transferred.") } reader.readAsBinaryString(file); } </script> </body> </html>
server side closed reason message is as below
socket closed: The decoded text message was too big for the output buffer and the endpoint does not support partial messages
Q1: It seems that it is finding text processing method instead of binary processing method according to the closed reason, how can I fix this?
Q2: Should I change data type to Blob to transfer file on javascript side? Then how?
extra Q: May anyone link example source(es) of websocket file transferring(java websocket or javascript either/both)?
Thanks for reading :)