File Upload using Twitter Bootstrap, C#, asp.net and javascript
Since you want to do this without a standard asp.net control, you will have to do some of the wiring that asp.net does for you.
Make sure your input has an id. I will set it here to myFile.
<div class="row-fluid">
<div class="fileupload fileupload-new" data-provides="fileupload"><input type="hidden">
<div class="input-append">
<div class="uneditable-input span2" runat="server" id="statment1">
<i class="icon-file fileupload-exists"></i>
<span class="fileupload-preview" style=""></span>
</div>
<span class="btn btn-file"><span class="fileupload-new">Select file</span>
<span class="fileupload-exists">Change</span><input id="myFile" type="file" runat="server">
</span>
<a href="#" class="btn fileupload-exists" data-dismiss="fileupload" >Remove</a>
</div>
</div>
</div>
Your page should now have a HtmlInputFile
control to your page. like this:
protected HtmlInputFile myFile;
Then you should be able to receive the file:
if (IsPostBack)
{
if (myFile.PostedFile != null)
{
// File was sent
var postedFile = myFile.PostedFile;
int dataLength = postedFile.ContentLength;
byte[] myData = new byte[dataLength];
postedFile.InputStream.Read(myData, 0, dataLength);
}
else
{
// No file was sent
}
}
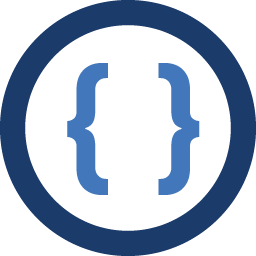
Admin
Updated on August 16, 2020Comments
-
Admin almost 4 years
link to Jasny http://jasny.github.com/bootstrap/javascript.html#fileupload
link to what the form looks like http://img507.imageshack.us/img507/3308/picpx.png
I am using the Jasny Javascript file upload in my boot strap project, it looks like this:
ASP\HTML VIEW
<div class="row-fluid"> <div class="fileupload fileupload-new" data-provides="fileupload"><input type="hidden"> <div class="input-append"> <div class="uneditable-input span2" runat="server" id="statment1"><i class="icon-file fileupload-exists"></i> <span class="fileupload-preview" style=""></span></div><span class="btn btn-file"><span class="fileupload-new">Select file</span><span class="fileupload-exists">Change</span><input type="file"></span><a href="#" class="btn fileupload-exists" data-dismiss="fileupload">Remove</a> </div> </div>
How do I go about using this in the code behind to save the attached file to my server as I would using the C# asp.net File Upload?
In ASP.net C# I would normally do this in the code behind:
ASP.net C# CodeBehind
string filename = FileUpload1.PostedFile.FileName; FileUpload1.PostedFile.SaveAs(Path.Combine(Server.MapPath("\\Document"), filename).ToString()); filelocation = "Document\\" + filename; media = "Document";
The Jasny github explains how to set the layout using bootstrap which is great as it looks really good (much better than the boring asp file upload) but How do I actually get I to post on my button click? I would really like to get this to work as I think it looks heaps nicer.