Fill DataGridView from SQLite DB (C#)
Solution 1
If you dont want to disturb the columns you can read the rows one by one using SQLiteDataReader and put it into the datagridview..
private void button1_Click_1(object sender, EventArgs e)
{
conn.Open();
SQLiteCommand comm = new SQLiteCommand("Select * From Patients", conn);
using (SQLiteDataReader read = comm.ExecuteReader())
{
while (read.Read())
{
dataGridView1.Rows.Add(new object[] {
read.GetValue(0), // U can use column index
read.GetValue(read.GetOrdinal("PatientName")), // Or column name like this
read.GetValue(read.GetOrdinal("PatientAge")),
read.GetValue(read.GetOrdinal("PhoneNumber"))
});
}
}
}
Solution 2
1) Set AutoGenerateColumns to false
2) dgv.Columns["Item"].DataPropertyName = "Item"; dgv.Columns["Quantity"].DataPropertyName = "Quantity";
3) Then instead of
select * from table1
use
select item Item,quantity Quantity from table1
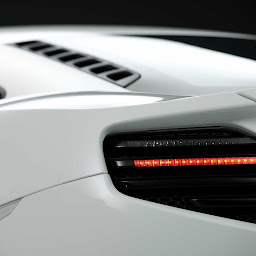
John
Updated on February 14, 2020Comments
-
John about 4 years
I'm trying to fill a datagridview from an SQLite database.
I've found plenty of ways of doing this. However, I have pre-existing columns in my dgv (Item, Quantity).
Currently, when I load the DB to the dgv, I get columns of the DB inserted into the dgv instead of the actual data being inserted into the correct column.
My SQLite DB, has a table with three columns (id PRIMARY KEY AUTO INCREMENT, item VARCHAR, quantity INTEGER).
How do load the DB into the pre-existing columns of the dgv?
Current code for filling dgv:
private void button1_Click_1(object sender, EventArgs e) { SetConnection(); sqlconnection.Open(); sqlcmd = sqlconnection.CreateCommand(); string CommandText = "SELECT * FROM table1"; sqlda = new SQLiteDataAdapter(CommandText, sqlconnection); using (dt = new DataTable()) { sqlda.Fill(dt); dataGridView1.DataSource = dt; }