Filter array of objects with another array of objects
Solution 1
var filtered = [];
for(var arr in myArray){
for(var filter in myFilter){
if(myArray[arr].userid == myFilter[filter].userid && myArray[arr].projectid == myFilter[filter].projectid){
filtered.push(myArray[arr].userid);
}
}
}
console.log(filtered);
Solution 2
You can put a couple of array methods to use here - filter
and some
. They're available in all recent browsers, and there are polyfills available for the older browsers.
const myArray = [{ userid: "100", projectid: "10", rowid: "0" }, { userid: "101", projectid: "11", rowid: "1"}, { userid: "102", projectid: "12", rowid: "2" }, { userid: "103", projectid: "13", rowid: "3" }, { userid: "101", projectid: "10", rowid: "4" }];
const myFilter = [{ userid: "101", projectid: "11" }, { userid: "102", projectid: "12" }, { userid: "103", projectid: "11"}];
const myArrayFiltered = myArray.filter((el) => {
return myFilter.some((f) => {
return f.userid === el.userid && f.projectid === el.projectid;
});
});
console.log(myArrayFiltered);
Solution 3
With Ecma script 6.
const myArrayFiltered = myArray.filter( el => {
return myfilter.some( f => {
return f.userid === el.userid && f.projectid === el.projectid;
});
});
Function:
const filterObjectArray = (arr, filterArr) => (
arr.filter( el =>
filterArr.some( f =>
f.userid === el.userid && f.projectid === el.projectid
)
)
);
console.log(filterObjectArray(myArray, myFilter))
Solution 4
In response to Andy answer above, which I believe should be marked now as answer., if you are looking for exact opposite behavior, use every with negation, something like this.
const result = masterData.filter(ad =>
filterData.every(fd => fd.userid !== md.userid));
result
contains all masterData
except filterData
.
Solution 5
based on @Renato his answer, but shorter:
const myArray = [{ userid: "100", projectid: "10", rowid: "0" }, ...];
const myFilter = [{ userid: "101", projectid: "11" }, ...];
const myArrayFiltered = myArray.filter(array => myFilter.some(filter => filter.userid === array.userid && filter.projectid === array.projectid));
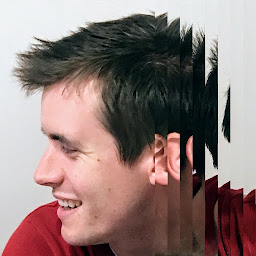
GtAntoine
React by day. React Native by night. UX developer by day and night ;)
Updated on July 09, 2022Comments
-
GtAntoine almost 2 years
This question is similar to this one Jquery filter array of object with loop but this time I need to do the filter with an array of objects.
Exemple:
I have an array of objects like this:
myArray = [ { userid: "100", projectid: "10", rowid: "0" }, { userid: "101", projectid: "11", rowid: "1"}, { userid: "102", projectid: "12", rowid: "2"}, { userid: "103", projectid: "13", rowid: "3" }, { userid: "101", projectid: "10", rowid: "4" } ...]
I want to filter it with an array like this:
myFilter = [ { userid: "101", projectid: "11" }, { userid: "102", projectid: "12" }, { userid: "103", projectid: "11" }]
and return this (the userid and the projectid in myFilter need to match the userid and the projectid in myArray):
myArrayFiltered = [ { userid: "101", projectid: "11", rowid: "1" }, { userid: "102", projectid: "12", rowid: "2" }]
How can I do that ?
-
NiallMitch14 over 4 yearsFantastic! Better than using a for loop! A lot more concise
-
TMA about 4 yearsHi @Andy, i am doing the same thing with the just one condition change about
not equal to !==
but that is not working in my case. So is there any other method to work with the not equal to condition ? Check my live example over here: playcode.io/586682 -
Ivar over 2 yearsDepending on your preference, you can also make the statement a bit more concise:
myArray.filter(el => myFilter.some(f => f.userid === el.userid && f.projectid === el.projectid))