Filtering combo box items using text search in C# windows form
12,932
Solution 1
you can add a textbox to your form and use it's text to filter :
string QueryCompany =
string.Format(
"select id,title from acc.dl where dltype in (2,4) union select null , null order by title");
SqlDataAdapter DA1 = new SqlDataAdapter(QueryCompany, con);
con.Open();
DataTable DT1 = new DataTable();
DA1.Fill(DT1);
con.Close();
DataView dv1 = new DataView(DT1);
dv1.RowFilter = "Title like '%" + txtCompany.Text + "%' or Title is null";
cmbCompany.DisplayMember = "Title";
cmbCompany.ValueMember = "id";
cmbCompany.DataSource = dv1;
and in selected index changed event :
txtCompany.Text = cmbCompany.Text;
Solution 2
Below part of code which works for me where searching part works not only at the begining but also in the middle. I paste also additional part responsible for move focus to the next control on the form after you hit enter button.
Initialization part:
public Form1()
{
InitializeComponent();
cmbItems = new List<ComboBoxItem>();
InitializeComboBox();
}
private void InitializeComboBox()
{
Random rand = new Random();
for (int i = 0; i <= 1500; i++)
{
int counter = rand.Next(1, 105000);
cmbItems.Add(new ComboBoxItem("randomNumber" + counter, "ID_" + counter));
}
comboBox1.DataSource = cmbItems;
}
Filtering part:
private void comboBox1_TextUpdate(object sender, EventArgs e)
{
if (comboBox1.Text == string.Empty)
{
comboBox1.DataSource = cmbItems; // cmbItems is a List of ComboBoxItem with some random numbers
comboBox1.SelectedIndex = -1;
}
else
{
string tempStr = comboBox1.Text;
IEnumerable<ComboBoxItem> data = (from m in cmbItems where m.Label.ToLower().Contains(tempStr.ToLower()) select m);
comboBox1.DataSource = null;
comboBox1.Items.Clear();
foreach (var temp in data)
{
comboBox1.Items.Add(temp);
}
comboBox1.DroppedDown = true;
Cursor.Current = Cursors.Default;
comboBox1.SelectedIndex = -1;
comboBox1.Text = tempStr;
comboBox1.Select(comboBox1.Text.Length, 0);
}
}
Moving focus part:
private void comboBox1_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar != '\r') return;
if (this.ActiveControl != null)
{
this.SelectNextControl(this.ActiveControl, true, true, true, true);
}
e.Handled = true; // Mark the event as handled
}
I hope that helps someone.
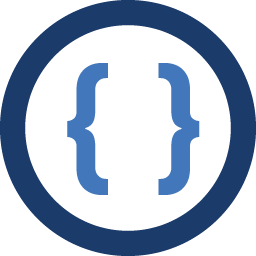
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I'm Trying to filter a combo box using its text property in all characters of Items not only Beginning of them. I'm trying below code in TextChanged event of my combo box. but unfortunately combo box resets after entering any key:
private void cmbCompany_TextChanged(object sender, EventArgs e) { string QueryCompany = string.Format("select id,title from acc.dl where title LIKE '%" + cmbCompany.Text + "%' union select null , null order by title"); SqlDataAdapter DA1 = new SqlDataAdapter(QueryCompany, con); DataTable DT1 = new DataTable(); DA1.Fill(DT1); cmbCompany.DisplayMember = "Title"; cmbCompany.ValueMember = "id"; cmbCompany.DataSource = DT1; }
connection string "con" is defined and opened. thanks for your helps.