Find all string matches with Regex golang
First, you do not need the regex delimiters. Second, it is a good idea to use raw string literals to define a regex pattern where you need to use only 1 backslash to escape regex metacharacters. Third, the capturing group is only necessary if you need to get the values without {
and }
, thus, you may remove it to get {city}
, {state}
and {zip}
.
You may use FindAllString
to get all matches:
r := regexp.MustCompile(`{[^{}]*}`)
matches := r.FindAllString("{city}, {state} {zip}", -1)
See the Go demo.
To only get the parts between curly braces use FindAllStringSubmatch
with a pattern that contains capturing parentheses, {([^{}]*)}
:
r := regexp.MustCompile(`{([^{}]*)}`)
matches := r.FindAllStringSubmatch("{city}, {state} {zip}", -1)
for _, v := range matches {
fmt.Println(v[1])
}
See this Go demo.
Regex details
-
{
- a literal{
char -
([^{}]*)
- a capturing group that matches any 0 or more (due to the*
quantifier) characters other than{
and}
([^...]
is a negated character class matching any char but the one(s) specified between[^
and]
) -
}
- a literal}
char
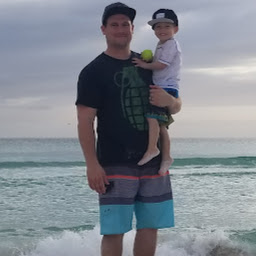
jrkt
Updated on July 09, 2022Comments
-
jrkt almost 2 years
I am trying to return an array, or slice, with all the matches for a specific regex expression against a string. The string is:
{city}, {state} {zip}
I want to return an array with all the matches of strings between curly braces. I have tried using the regexp package to accomplish this but cannot figure out how to return what I am looking for. This is my current code:
r := regexp.MustCompile("/({[^}]*})/") matches := r.FindAllString("{city}, {state} {zip}", -1)
But, all it returns is an empty slice every time no matter what I try.