Find phrase in string with preg_match
Solution 1
If you want to check if a sub-string is present in a string, no need for regular expressions : stripos()
will do just fine :
if (stripos(strtolower($line), 'see details') !== false) {
// 'see details' is in the $line
}
stripos()
will return the position of the first occurrence of the sub-string in the string ; or false
if the sub-string is not found.
Which means that if it returns something else than false
, the sub-string is found.
Solution 2
Your regex is actually broken.
/^(\see details)/
This breaks down into:
- At the beginning
- Open a capturing group
- Look for one whitespace character
- Followed by all of the following characters:
ee details
- Close the group
\s
is an escape sequence matching whitespace. You could also have added the i
modifier to make the regex case-insensitive. You also don't seem to be doing anything with the captured group, so you can ditch that.
Therefore:
/^see details/i
is what you'd want.
You mentioned that you're going through input line by line. If you only need to know that the entire input contains the specific string, and you have the input as a string, you can use the m
modifier to make ^
match "beginning of a line" instead of / in addition to "beginning of the string":
/^see details/im
If this is the case, then you would end up with:
if(preg_match('/^see details/im', $whole_input)) {
echo "See Details Found!";
}
But as others have mentioned, a regex isn't needed here. You can (and should) do the job with the more simple stripos
.
Solution 3
As Pascal said, you can use stripos()
function although correct code would be:
if (stripos(strtolower($line), 'see details') !== false) {
// 'see details' is in the $line
}
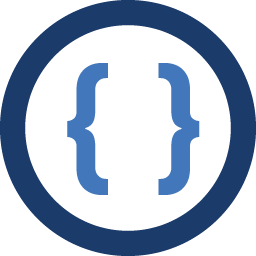
Admin
Updated on July 05, 2022Comments
-
Admin almost 2 years
I am searching through text line by line and want to see if the line contains the phrase "see details" and is not case sensitive, so will find:
See Details, See details, SEE Details etc
I have this so far.
if(preg_match("/^(\see details)/", strtolower($line))) { echo 'SEE DETAILS FOUND'; }
A simple example would be helpful thanks.
-
Admin about 13 yearsThanks makes more sense to do it that way. 1st line should be if (strpos(strtolower($line), 'see details') !== false).
-
Pascal MARTIN about 13 yearsoops ^^ yes, of course :-) sorry about that, forgot to type half of it... (I've edited my answer to fix that ; thanks for the comment !)
-
knittl about 13 yearsno need to use
strtolower
when already using case insensitive matching (stripos
)