Find specific word in text file and count it
100,653
Solution 1
public int countWord(String word, File file) {
int count = 0;
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String nextToken = scanner.next();
if (nextToken.equalsIgnoreCase(word))
count++;
}
return count;
}
Solution 2
HashMap h=new HashMap();
FileInputStream fin=new FileInputStream("d:\\file.txt");
BufferedReader br=new BufferedReader(new InputStreamReader(fin));
String n;
while((n=br.readLine())!=null)
{
if(h.containsKey(n))
{
int i=(Integer)h.get(n);
h.put(n,(i+1));
}
else
h.put(n, 1);
}
now iterate through this map to get the count for each word using each word as a key to the map values
Solution 3
Apache Commons - StringUtils.countMatches()
Solution 4
package somePackage;
public static void main(String[] args) {
String path = ""; //ADD YOUR PATH HERE
String fileName = "test2.txt";
String testWord = "Macbeth"; //CHANGE THIS IF YOU WANT
int tLen = testWord.length();
int wordCntr = 0;
String file = path + fileName;
boolean check;
try{
FileInputStream fstream = new FileInputStream(file);
BufferedReader br = new BufferedReader(new InputStreamReader(fstream));
String strLine;
//Read File Line By Line
while((strLine = br.readLine()) != null){
//check to see whether testWord occurs at least once in the line of text
check = strLine.toLowerCase().contains(testWord.toLowerCase());
if(check){
//get the line, and parse its words into a String array
String[] lineWords = strLine.split("\\s+");
for(String w : lineWords){
//first see if the word is as least as long as the testWord
if(w.length() >= tLen){
/*
1) grab the specific word, minus whitespace
2) check to see whether the first part of it having same length
as testWord is equivalent to testWord, ignoring case
*/
String word = w.substring(0,tLen).trim();
if(word.equalsIgnoreCase(testWord)){
wordCntr++;
}
}
}
}
}
System.out.println("total is: " + wordCntr);
//Close the input stream
br.close();
} catch(Exception e){
e.printStackTrace();
}
}
Solution 5
public class Wordcount
{
public static void main(String[] args)
{
int count=0;
String str="hi this is is is line";
String []s1=str.split(" ");
for(int i=0;i<=s1.length-1;i++)
{
if(s1[i].equals("is"))
{
count++;
}
}
System.out.println(count);
}
}
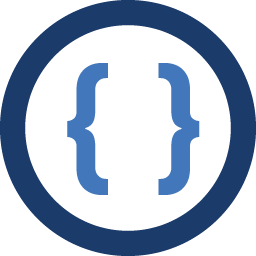
Author by
Admin
Updated on January 12, 2020Comments
-
Admin over 4 years
someone can help me with code? How to search in text file any word and count how many it were repeated?
For example test.txt:
hi hola hey hi bye hoola hi
And if I want to know how many times are repeated in test.txt word "Hi" program must say "3 times repeated"
I hope you understood what I want, thank you for answers.