Find string within JSON with Python
Solution 1
date['device']
contains a list of objects, so you should treat it as such and iterate over them:
for element in data['device']:
if element['serial'] == '00000000762c1d3c':
print 'there'
print element['registered_id']
break
else:
print 'not there'
This is using the somehow lesser-known for-else
construct: https://docs.python.org/3/tutorial/controlflow.html#break-and-continue-statements-and-else-clauses-on-loops
Solution 2
first, your input isn't json. Json uses double quotes. But suppose you successfully loaded it with json, it's now a dictionary, called d
.
Then you can scan all sub-dicts of d
and test serial
key against your value, stopping when found using any
and a generator comprehension:
print(any(sd['serial']=='00000000762c1d3c' for sd in d['device']))
returns True
if serial found False
otherwise.
Related videos on Youtube
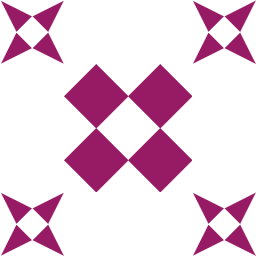
user5740843
Updated on May 15, 2022Comments
-
user5740843 13 days
I'm currently getting a very long JSON and I'm trying to pick out 2 pieces of information from it through Python 2.7.
The JSON looks roughly like this:
{ 'device': [ { 'serial': '00000000762c1d3c', 'registered_id': '019' }, { 'serial': '000000003ad192f2', 'registered_id': '045' }, { 'serial': '000000004c9898aa', 'registered_id': '027' } ], }
Within this JSON I'm looking for a specific serial that might match with one in the JSON. If it does, it should print out the registered_id as well.
I've tried using a simple script, even without the registered_id but I'm getting nowhere.:
if '00000000762c1d3c' not in data['device']: print 'not there' else: print 'there'
Thanks for your suggestions!