Find the biggest number in an array by using JavaScript loops
Solution 1
zer00ne's answer should be better for simplicity, but if you still want to follow the for-loop way, here it is:
function biggestNumberInArray (arr) {
// The largest number at first should be the first element or null for empty array
var largest = arr[0] || null;
// Current number, handled by the loop
var number = null;
for (var i = 0; i < arr.length; i++) {
// Update current number
number = arr[i];
// Compares stored largest number with current number, stores the largest one
largest = Math.max(largest, number);
}
return largest;
}
Solution 2
Some ES6 magic for you, using the spread syntax:
function biggestNumberInArray(arr) {
const max = Math.max(...arr);
return max;
}
Actually, a few people have answered this question in a more detailed fashion than I do, but I would like you to read this if you are curious about the performance between the various ways of getting the largest number in an array.
Solution 3
There are multiple ways.
- Using Math max function
let array = [-1, 10, 30, 45, 5, 6, 89, 17];
console.log(Math.max(...array))
- Using reduce
let array = [-1, 10, 30, 45, 5, 6, 89, 17];
console.log(array.reduce((element,max) => element > max ? element : max, 0));
- Implement our own function
let array = [-1, 10, 30, 45, 5, 6, 89, 17];
function getMaxOutOfAnArray(array) {
let maxNumber = -Infinity;
array.forEach(number => { maxNumber = number > maxNumber ? number : maxNumber; })
console.log(maxNumber);
}
getMaxOutOfAnArray(array);
Related videos on Youtube
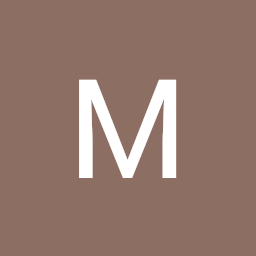
Jacky
Updated on April 06, 2022Comments
-
Jacky about 2 years
Create a function called biggestNumberInArray().
That takes an array as a parameter and returns the biggest number.
Here is an array
const array = [-1, 0, 3, 100, 99, 2, 99]
What I try in my JavaScript code:
function biggestNumberInArray(arr) { for (let i = 0; i < array.length; i++) { for(let j=1;j<array.length;j++){ for(let k =2;k<array.length;k++){ if(array[i]>array[j] && array[i]>array[k]){ console.log(array[i]); } } } } }
It returns 3 100 99.
I want to return just 100 because it is the biggest number.
Is there a better way to use loops to get the biggest value?
Using three different JavaScript loops to achieve this (for, forEach, for of, for in).
You can use three of them to accomplish it.
-
zer00ne about 5 years
Math.max.apply({}, array);
-
Wyck about 5 yearsOr search the web for "Kth largest element". e.g.: geeksforgeeks.org/kth-smallestlargest-element-unsorted-array This is typically an interview question.
-
cegfault about 5 yearsThere is no need for nested for loops here. Hint: use a variable to store the max value
-
zer00ne about 5 yearsSure you can put anything in a loop, real question is should you?
-
Wyck about 5 years@cegfault, this is typically the precursor question to getting an interview candidate to implement a kth largest element algorithm or to talk about order statistics. So using max only helps you with the first part. The next part is always how would you find the 2nd largest, or top-2. Then 3, then k.
-
cegfault about 5 years... to getting an interview candidate .... ah. got it
-
Jacky about 5 yearsOk,I'll try to put Math.max.apply in loops
-
holydragon about 5 yearsYou also can sort the array and then pop it.
-
Jacky about 5 yearsOk,I found the answer...sorry,guys.Can i delete this question?
-
-
wentjun about 5 yearsThanks for pointing that out lol, I have must have mistyped it. But yeah I have edited it that very instant.
-
Andy about 5 yearsBut why use the spread syntax here? What does it do, and how is that related to how
Math.max
reads numbers? -
Andy about 5 yearsWhat does
apply
do, and how does it relate to howMath.max
works? The OP probably doesn't know so you should include that information in your answer. -
Adrian Brand about 5 yearsThe spread operator turns the array into a list of parameters, max doesn't take an array as the argument.
-
wentjun about 5 yearsHonestly I dont mean to overcomplicate things! I suggested using the spread syntax because no one else at that point of time has suggested using it.
-
Adrian Brand about 5 yearsReduce is much more performant jsperf.com/max-vs-reduce/1
-
RobG about 5 years
var a = [1,3,2]; for (var max=a[0], i=1, iLen=a.length; i<iLen; i++) if (a[i]>max) max=a[i];
. -
Peter Mortensen almost 4 yearsWho is "zer00ne"? Based on the time of posting, it could refer to any of the answers here (including the deleted one).
-
Matheus Pratta almost 4 years@PeterMortensen I have linked it here just in case.
-
Sebastian Simon over 3 years
-
Trilok Singh over 3 yearsWe want to find a big number. This approach is wrong?
-
Sebastian Simon over 3 years“This approach is wrong?” — It’s not the approach demanded in the question, so yes this approach is wrong in this context. When answering, please make sure to answer the question, not just the question title. And please don’t repeat other answers. At least format your code.
-
Trilok Singh over 3 yearsAnd please don’t repeat other answers . means ?
-
Admin over 2 yearsYour answer could be improved with additional supporting information. Please edit to add further details, such as citations or documentation, so that others can confirm that your answer is correct. You can find more information on how to write good answers in the help center.
-
General Grievance about 2 yearsIf all elements are negative, this will not work.