Findbugs android gradle plugin
Solution 1
Just place this in your modules build.gradle
.
apply plugin: 'findbugs'
task customFindbugs(type: FindBugs) {
ignoreFailures = false
effort = "max"
reportLevel = "low"
classes = files("$project.buildDir/intermediates/classes")
// Use this only if you want exclude some errors
excludeFilter = file("$rootProject.rootDir/config/findbugs/exclude.xml")
source = fileTree('src/main/java/')
classpath = files()
reports {
xml.enabled = false
xml.withMessages = true
html.enabled = !xml.isEnabled()
xml.destination "$project.buildDir/outputs/findbugs/findbugs-output.xml"
html.destination "$project.buildDir/outputs/findbugs/findbugs-output.html"
}
}
build.dependsOn customFindbugs
Then after changing directory to your project path from command line, use
./gradlew build
The error report will be in $project.buildDir/outputs/findbugs/findbugs-output.html
Solution 2
I modified a little bit Nevin Raj Victor's answer.
This version generates a findbug task for each build variant, and (more importantly) it correctly creates dependencies on their respective compilation tasks. Indeed, findbugs requires the code to be compiled before it can be analyzed.
// findbug tasks for each variant
apply plugin: 'findbugs'
android.applicationVariants.all { variant ->
task("findbugs${variant.name.capitalize()}", type: FindBugs) {
description "Analyze ${variant.name} code with the findbugs tool"
group "Verification"
ignoreFailures = true
effort = "default"
reportLevel = "medium"
classes = files("$project.buildDir/intermediates/classes/${variant.dirName}")
excludeFilter = file("$rootProject.rootDir/findbugs/findbugs-filter.xml")
source = variant.javaCompile.source
classpath = variant.javaCompile.classpath
reports {
// Only one of HTML or XML can be turned on at the same time
html.enabled = true
xml.enabled = !html.enabled
xml.withMessages = true
html.destination = "$project.buildDir/outputs/findbugs/findbugs-${variant.name}-output.html"
xml.destination = "$project.buildDir/outputs/findbugs/findbugs-${variant.name}-output.xml"
}
dependsOn "compile${variant.name.capitalize()}JavaWithJavac"
}
}
After this, you can run
./gradlew findbugsDebug
./gradlew findbugsRelease
Or other findbugs tasks on different variants, depending on your configuration.
Solution 3
Please take a look at this project https://github.com/Piasy/AndroidCodeQualityConfig.
This project including lint, pmd, findbugs, checkstyle, jacoco code coverage.And support project with submodules.
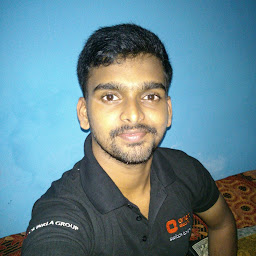
Nevin Raj Victor
Working as Configuration Manager in a well reputed IT company. Have knowledge in continuous integration tool like TeamCIty for software deployment. Also have some knowledge in version control. Working on to get deeper on scripting languages like python and bash.
Updated on July 22, 2022Comments
-
Nevin Raj Victor almost 2 years
I have an android project. I want to introduce
findbugs
in my project as a gradle plugin. I tried to edit the project'sbuild.gradle
as below.buildscript { repositories { mavenCentral() maven { url 'https://maven.fabric.io/public' } } dependencies { classpath 'com.android.tools.build:gradle:1.0.0+' classpath 'io.fabric.tools:gradle:1.+' } } apply plugin: "java" apply plugin: "findbugs" findbugs { toolVersion = "2.0.1" sourceSets = [sourceSets.main] ignoreFailures = false reportsDir = file("$project.buildDir/findbugsReports") effort = "max" reportLevel = "high" includeFilter = file("$rootProject.projectDir/config/findbugs/includeFilter.xml") excludeFilter = file("$rootProject.projectDir/config/findbugs/excludeFilter.xml") }
Is this plugin correct? Does anything neeed to be added or removed? Now what should I do to get the results of this
findbugs
check? What gradle command should I use?