finding an element from one list in another list in python
Solution 1
Looking up the position of an item in a list is not a very efficient operation. A dict is a better data structure for this kind of task.
>>> d = {k:v for v,k in enumerate(list_one)}
>>> print(*(d[k] for k in list_two))
8 5 12 12 15
If your list_one
is always just the alphabet, in alphabetical order, it's probably better and simpler to get something working by using the builtin function ord
.
Solution 2
Adding to @wim's answer, could be done with a simple comprehension.
>>> [list_one.index(x) for x in list_two]
[8, 5, 12, 12, 15]
Solution 3
you can iterate thought the list :
>>> for i in range(len(list_two)):
... for j in range(len(list_one)):
... if list_two[i]==list_one[j]:
... list_3.append(j)
>>> list_3
[8, 5, 12, 12, 15]
but wim's answer is more elegant !
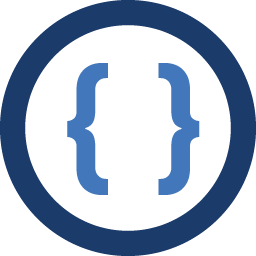
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Is there a way to have two lists named list1 and list2 and be able to look up the position of one entry in another. i.e.
list_one = ["0", "a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v", "w", "x", "y", "z"] list_two = ["h","e","l","l","o"]
my aim is to allow the user to enter a word which the program will then convert in a set of numbers corresponding to the letters entries in list_one
so if the user did input hello the computer would return 85121215 (being the position of the entries)
is there a possible way to do this
-
Admin about 7 yearsthanks! this also helps me. I was looking for a way to store the output as a variable and yours does that. thanks!
-
Astrid over 5 yearsThis is much cleaner.