Finding minimum and maximum in Java 2D array
Solution 1
Ok, I've kinda fixed your code. Actually your mistake was that you have not been traversing all the cells of your multidimensional array.
So, I've added additional loop into getMinValue/getMinValue methods and fixed array elements addressing.
import java.util.*;
class MinMax {
public static void main(String[] args) {
int[][] data = {
{3, 2, 5},
{1, 4, 4, 8, 13},
{9, 1, 0, 2},
{0, 2, 6, 3, -1, -8}
};
System.out.println(getMaxValue(data));
System.out.println(getMinValue(data));
}
public static int getMaxValue(int[][] numbers) {
int maxValue = numbers[0][0];
for (int j = 0; j < numbers.length; j++) {
for (int i = 0; i < numbers[j].length; i++) {
if (numbers[j][i] > maxValue) {
maxValue = numbers[j][i];
}
}
}
return maxValue;
}
public static int getMinValue(int[][] numbers) {
int minValue = numbers[0][0];
for (int j = 0; j < numbers.length; j++) {
for (int i = 0; i < numbers[j].length; i++) {
if (numbers[j][i] < minValue ) {
minValue = numbers[j][i];
}
}
}
return minValue ;
}
}
Solution 2
I have a more fun solution using Java 8 :)
IntSummaryStatistics stats = Arrays.stream(data).flatMapToInt(Arrays::stream).collect(Collectors.summarizingInt(Integer::intValue));
int max = stats.getMax();
int min = stats.getMin();
It's a different solution than yours, obviously. But it does the same thing. To begin with, we convert the 2D array into a Stream
of int
s. In order to do this first we need to call flatMapToInt
. We do this to stream all the elements in the array in a flat way. Imagine if we just start using a single index to iterate over the whole 2D array. It's something like that. Once we have converted the array into a stream, we will use IntSummaryStatistics in order to reuse the same stream for both min and max.
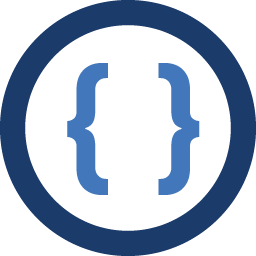
Admin
Updated on December 27, 2020Comments
-
Admin over 3 years
I have been trying to figure this out for a while and need some help. I need to find the min/max values and print them out for a multidimensional array. Here are the two ways that I have tried.
import java.util.*; class MinMax { public static void main(String[] args) { int[][] data = {{3, 2, 5}, {1, 4, 4, 8, 13}, {9, 1, 0, 2}, {0, 2, 6, 3, -1, -8}}; Arrays.sort(data); System.out.println("Minimum = " + data[0]); System.out.println("Maximum = " + data[data.length - 1]); } }
This version complies but doesn't run.
import java.util.*; class MinMax { public static void main(String[] args) { int[][] data = {{3, 2, 5}, {1, 4, 4, 8, 13}, {9, 1, 0, 2}, {0, 2, 6, 3, -1, -8}}; public static int getMaxValue(int[] numbers) { int maxValue = numbers[0]; for (int i = 1; i < numbers.length; i++) { if (numbers[i] > maxValue) { maxValue = numbers[i]; } return maxValue; { public static int getMinValue (int[] numbers) { int minValue = numbers[0]; for (int i = 1; i < numbers.length; i++) { if (numbers[i] < minValue) { minValue = numbers[i]; } } return minValue; }
This version just throws me a bunch of errors in compiling. Any help is greatly appreciated.
-
bcsb1001 almost 8 yearsMethod references are fun:
x -> Arrays.stream(x)
can be simplified toArrays::stream
. -
Alexander Petrov almost 8 yearsYou know what I find really funny. Once I started doing the Java8 thing , some things are just soo... ridiculously simple and require so much less code. I wonder how have we coded before Java 8 ?
-
Admin almost 8 yearsOh okay I see. Thank you so much! I've been pulling my hairs out on this one
-
Admin almost 8 yearsMan that seems so much simpler! I suppose once I get through the beginners stuff i get to have some more fun in the future haha!
-
Admin almost 8 yearsI was able to figure out how to find the values using a 1D array, thanks for the detail on how to declare it for a 2D array!
-
Sebastian S over 5 yearsPlease don't just post code, but explain how this answers the question.
-
Alexander Petrov almost 5 yearsThere was a small error in the example that I corrected through the usage of IntSummaryStatistics. @Fanchen Bao pointed out a stream can be consumed only once. Therefore we use IntSummaryStatistics in order to avoid recreating the same stream for min and max.
-
luckyguy73 almost 4 yearsthis code didn't work for me, however, a small change and it works by using this: Arrays.stream(gameField).flatMapToInt(Arrays::stream).summaryStatistics();
-
Carlos Medina about 3 years@luckyguy73 this really helped, not sure how Alex got his code to work as I had your same problem.