Finding total contributions of a user from Github API
Solution 1
Answers for 2019, Use GitHub API V4.
First go to GitHub to apply for a token: https://help.github.com/en/github/authenticating-to-github/creating-a-personal-access-token-for-the-command-line. step 7, scopes select only read:user
cUrl
curl -H "Authorization: bearer token" -X POST -d '{"query":"query {\n user(login: \"MeiK2333\") {\n name\n contributionsCollection {\n contributionCalendar {\n colors\n totalContributions\n weeks {\n contributionDays {\n color\n contributionCount\n date\n weekday\n }\n firstDay\n }\n }\n }\n }\n}"}' https://api.github.com/graphql
JavaScript
async function getContributions(token, username) {
const headers = {
'Authorization': `bearer ${token}`,
}
const body = {
"query": `query {
user(login: "${username}") {
name
contributionsCollection {
contributionCalendar {
colors
totalContributions
weeks {
contributionDays {
color
contributionCount
date
weekday
}
firstDay
}
}
}
}
}`
}
const response = await fetch('https://api.github.com/graphql', { method: 'POST', body: JSON.stringify(body), headers: headers })
const data = await response.json()
return data
}
const data = await getContributions('token', 'MeiK2333')
console.log(data)
Solution 2
Yes, You can do this easily with the new graphql API
Check out the explorer: https://developer.github.com/v4/explorer/
There you can see the contributions collection which is an edge of the user. You can get all of the information necessary to rebuild the calendar.
I've included a full example, and the explorer documentation can guide you even further.
Specifically to answer your question, the query.user.contributionsCollection.contributionsCalendar.totalContributions
is what you are looking for
Go ahead and copy/paste the following into the explorer and you will see my contribution history for the last year
query {
user(login: "qhenkart") {
email
createdAt
contributionsCollection(from: "2019-09-28T23:05:23Z", to: "2020-09-28T23:05:23Z") {
contributionCalendar {
totalContributions
weeks {
contributionDays {
weekday
date
contributionCount
color
}
}
months {
name
year
firstDay
totalWeeks
}
}
}
}
}
Solution 3
You can get the svg calendar from https://github.com/users/<USER>/contributions
with to
URL parameter like :
https://github.com/users/bertrandmartel/contributions?to=2016-12-31
You can use a basic xml parser to sum all the contributions from the svg.
An example with curl & xmlstarlet for year 2016:
curl -s "https://github.com/users/bertrandmartel/contributions?to=2016-12-31" | \
xmlstarlet sel -t -v "sum(//svg/g/g/rect/@data-count)"
Solution 4
You can use the github events api for that:
Example (node.js)
const got = require('got')
async function getEvents(username) {
const events = []
let page = 1
do {
const url = `https://api.github.com/users/${username}/events?page=${page}`
var { body } = await got(url, {
json: true
})
page++
events.push(...body)
} while(!body.length)
return events
}
(async () => {
const events = await getEvents('handtrix')
console.log('Overall Events', events.length)
console.log('PullRequests', events.filter(event => event.type === 'PullRequestEvent').length)
console.log('Forks', events.filter(event => event.type === 'ForkEvent').length)
console.log('Issues', events.filter(event => event.type === 'IssuesEvent').length)
console.log('Reviews', events.filter(event => event.type === 'PullRequestReviewEvent').length)
})()
Example (javascript)
async function getEvents(username) {
const events = []
let page = 1
do {
const url = `https://api.github.com/users/${username}/events?page=${page}`
var body = await fetch(url).then(res => res.json())
page++
events.push(...body)
} while(!body.length)
return events
}
(async () => {
const events = await getEvents('handtrix')
console.log('Overall Events', events.length)
console.log('PullRequests', events.filter(event => event.type === 'PullRequestEvent').length)
console.log('Forks', events.filter(event => event.type === 'ForkEvent').length)
console.log('Issues', events.filter(event => event.type === 'IssuesEvent').length)
console.log('Reviews', events.filter(event => event.type === 'PullRequestReviewEvent').length)
})()
Documentation
- https://developer.github.com/v3/activity/events/
- https://developer.github.com/v3/activity/events/types/
- https://www.npmjs.com/package/got
Solution 5
To load the svg with all contributions you can use this code in your html page
<img src="https://ghchart.rshah.org/username" alt="Name Your Github chart">
To customize color you can just do that
<img src="https://ghchart.rshah.org/HEXCOLORCODE/username" alt="Name Your Github chart">
Related videos on Youtube
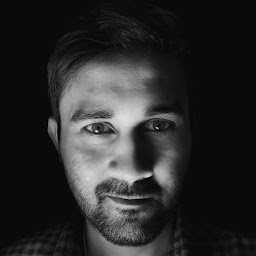
AnkitG
I am working with realtor.com, A Ruby and Ruby on Rails enthusiast and a Rails Contributor, Agile and a scrum practitioner and a Photographer. Creator of followg gems: Carrierwave Encrypter Decrypter Days Picker Saturday HighStocks rails Piecon Rails Font Awesome icons list
Updated on September 15, 2022Comments
-
AnkitG over 1 year
I am trying to extract the below info for any user from github.
Is there a way/api exposed in github-api where we can get this information directly ?
-
Nef10 over 4 yearsWhen using this keep in mind that it only returns data from the last 90 days
-
hakatashi over 3 yearsFYI: If you only want to know the total contribution of the authenticated user,
{"query": "query {viewer {contributionsCollection {contributionCalendar {totalContributions}}}}"}
should be enough. -
Anoushk almost 3 yearsCan you explain why you have used method POST, shouldn't it be GET?
-
YuliaP stands with Ukraine almost 3 years@Maverick it is POST b/c graphQL is being used for this request. see this link - stackoverflow.com/questions/59162265/…
-
lkahtz over 2 yearsLink seems broken now.