Firebase cloud function returns null in Flutter because it's still running
Solution 1
Callable functions must return a promise that resolves with the data to send to the client. As shown, the function isn't returning anything at the top level. Returning from the then
callback isn't sufficient. You will also need to return the promise returned by then
:
export const pubVer = functions.https.onCall((data, context) => {
console.log(data.message);
const kRef = admin.firestore().collection('Keys').doc(data.message)
return kRef.get()
.then(doc => {
if (!doc.exists) {
console.log('No such document!');
return {returnMessage: 'FALSE'}
} else {
console.log('DOC DOES EXIST');
return {'returnMessage: 'TRUE'}
}
})
.catch(err => {
console.log('Error getting document', err);
});
});
Solution 2
I recommend using async/await that could help you debug
export const pubVer = functions.https.onCall(async (data, context) => {
const documentId = data.message;
const kRef = admin.firestore().collection('Keys').doc(documentId);
try {
const doc = await kRef.get();
if (!doc.exists)
throw new Error('No such document!');
return doc.data();
} catch (e) {
console.log(e);
return null;
}
});
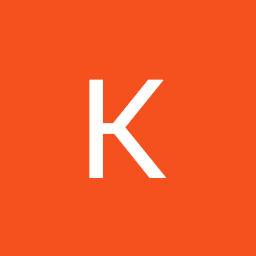
Karel Debedts
Updated on December 13, 2022Comments
-
Karel Debedts over 1 year
UPDATE: I'm getting this out my firebase console: Function execution took 2906 ms, finished with status code: 200 And 15 seconds later, I get: the console.log DOC DOES EXIST
I'm running this cloud firestore function. It 'works' but I got constantly this as a return: FLUTTER: NULL
But within the firebase console I get the console.log that says 'DOC DOES EXIST'.
What could be the solution to this? Language TYPESCRIPT
Thank you very much!
export const pubVer = functions.https.onCall((data, context) => { console.log(data.message); const kRef = admin.firestore().collection('Keys').doc(data.message) kRef.get() .then(doc => { if (!doc.exists) { console.log('No such document!'); return {returnMessage: 'FALSE'} } else { console.log('DOC DOES EXIST'); return {'returnMessage: 'TRUE'} } }) .catch(err => { console.log('Error getting document', err); }); });
-
Karel Debedts over 4 yearsThank you! When returning doc.data(), I'm trying to set a new document in firestore with the users ID and mailadress. But when I try to implement it: it says : "Promises must be handled appropriately"
-
EdHuamani over 4 years@KarelDebedts Can you share your complete function?