Firebase Remote Config fetch doesn't update values from the Cloud
Solution 1
For development testing, specify a cache expiration time of zero to force an immediate fetch:
mFirebaseRemoteConfig.fetch(0) // <- add the zero
.addOnCompleteListener(this, new OnCompleteListener<Void>() {
...
});
Solution 2
Some tips the helped to me:
- Don't forget to click
"publish changes"
in Firebase console after each value update - Uninstall and install the App before checking (Firebase may not fetch immediately)
- Use
mFirebaseRemoteConfig.fetch(0)
Solution 3
For what it worth I found that our firebase remote configs weren't downloading no matter what we tried - we are usually debugging while connected to a proxy (like Charles Proxy) and that was interrupting the firebase cloud update.
Once we connected to a non-proxied wifi connection we got the update.
You can also set your config to developer mode if running a debug build which will refresh values more often - but the proxy was our root problem.
FirebaseRemoteConfigSettings configSettings = new FirebaseRemoteConfigSettings.Builder()
.setDeveloperModeEnabled(BuildConfig.DEBUG)
.build();
Solution 4
In Flutter, I just changed the value of minimumFetchInterval to const Duration(hours: 0). I manually added an message to firebase and here I just get it. Here is my code;
await Firebase.initializeApp();
RemoteConfig remoteConfig = RemoteConfig.instance;
await remoteConfig.setConfigSettings(RemoteConfigSettings(
fetchTimeout: const Duration(seconds: 10),
minimumFetchInterval: const Duration(hours: 0),
));
RemoteConfigValue(null, ValueSource.valueStatic);
bool updated = await remoteConfig.fetchAndActivate();
if (updated) {
print("it is updated");
} else {
print("it is not updated");
}
print('my_message ${remoteConfig.getString('my_message')}');
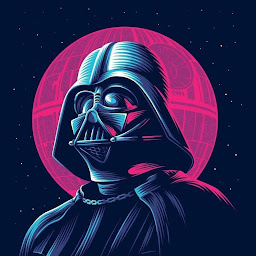
Comments
-
Furetur about 2 years
I'm trying to set up Firebase Remote Config for my project. I added Firebase via the Assistant. I added values to the server values on Google Cloud Console:
I've created default values xml in res/xml
<defaultsMap> <!-- Strings--> <entry > <key>textView_send_text</key> <value >your phrase goes here.</value> </entry> </defaultsMap>
Thats my MainActivity:
final private FirebaseRemoteConfig mFirebaseRemoteConfig = FirebaseRemoteConfig.getInstance(); protected void onCreate(Bundle savedInstanceState) { //..code.. //fetch from Firebase fetchAll(); } private void fetchAll(){ final FirebaseRemoteConfig mFirebaseRemoteConfig = FirebaseRemoteConfig.getInstance(); FirebaseRemoteConfigSettings configSettings = new FirebaseRemoteConfigSettings.Builder() .setDeveloperModeEnabled(BuildConfig.DEBUG) .build(); mFirebaseRemoteConfig.setConfigSettings(configSettings); mFirebaseRemoteConfig.setDefaults(R.xml.defaults); mFirebaseRemoteConfig.fetch() .addOnCompleteListener(this, new OnCompleteListener<Void>() { @Override public void onComplete(@NonNull Task<Void> task) { if(task.isSuccessful()){ Toast.makeText(MainActivity.this, "Fetch Succeeded", Toast.LENGTH_SHORT).show(); mFirebaseRemoteConfig.activateFetched(); }else{ Toast.makeText(MainActivity.this, "Fetch Failed", Toast.LENGTH_SHORT).show(); } displayWelcomeMessage(); } }); } private void displayWelcomeMessage(){ String welcomeMessage = mFirebaseRemoteConfig.getString("textView_send_text"); Toast.makeText(this, welcomeMessage, Toast.LENGTH_SHORT).show(); }
Toast output:
So Toast gets the value from xml/defaults not from the Cloud. It'd be much appreciated if somebody found where I made a mistake.
-
j2emanue almost 7 yearsdont do it too many times or you'll have a exception. i recommend the emulator so you can switch a few times if you reach the throttle limit.
-
CoolMind over 5 yearsThough it solves a problem, after several launches an application stops getting
OnCompleteListener
events. -
flopshot over 5 yearsWere you ever able to find a solution for remote config while proxying? We were already enabling developer mode.
-
Arpit J. over 4 yearssetDeveloperModeEnabled(boolean enabled) is deprecated now.
-
NSExceptional over 4 yearsPoint 2 was the thing I was missing, worked like a charm on iOS
-
4ntoine over 3 yearsReinstalling also worked for me. Can you please explain why reinstall is required? How can i make sure it is indeed updated without reinstall in production?
-
Pavel Poley over 3 years@4ntoine reinstall required when you testing your app, since you don't want to wait. remote config will be updated in production, but not immediately