Firebase TIMESTAMP to date and Time
Solution 1
timestamp is an object
timestamp= {nanoseconds: 0,
seconds: 1562524200}
console.log(new Date(timestamp.seconds*1000))
Solution 2
Firebase.ServerValue.TIMESTAMP is not actual timestamp it is constant that will be replaced with actual value in server if you have it set into some variable.
mySessionRef.update({ startedAt: Firebase.ServerValue.TIMESTAMP });
mySessionRef.on('value', function(snapshot){ console.log(snapshot.val()) })
//{startedAt: 1452508763895}
if you want to get server time then you can use following code
fb.ref("/.info/serverTimeOffset").on('value', function(offset) {
var offsetVal = offset.val() || 0;
var serverTime = Date.now() + offsetVal;
});
Solution 3
For those looking for the Firebase Firestore equivalent. It's
firebase.firestore.FieldValue.serverTimestamp()
e.g.
firebase.firestore().collection("cities").add({
createdAt: firebase.firestore.FieldValue.serverTimestamp(),
name: "Tokyo",
country: "Japan"
})
.then(function(docRef) {
console.log("Document written with ID: ", docRef.id);
})
.catch(function(error) {
console.error("Error adding document: ", error);
});
Solution 4
In fact, it only work to me when used
firebase.database.ServerValue.TIMESTAMP
With one 'database' more on namespace.
Solution 5
inside Firebase Functions transform the timestamp like so:
timestampObj.toDate()
timestampObj.toMillis().toString()
documentation here https://firebase.google.com/docs/reference/js/firebase.firestore.Timestamp
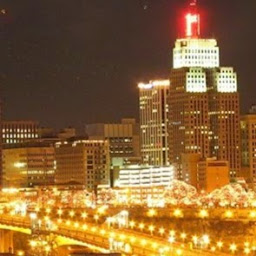
Kichu
Updated on July 05, 2022Comments
-
Kichu almost 2 years
I am using firebase for my chat application. In chat object I am adding time stamp using
Firebase.ServerValue.TIMESTAMP
method.I need to show the message received time in my chat application using this Time stamp .
if it's current time i need to show the time only.It's have days difference i need to show the date and time or only date.
I used the following code for convert the Firebase time stamp but i not getting the actual time.
var timestamp = '1452488445471'; var myDate = new Date(timestamp*1000); var formatedTime=myDate.toJSON();
Please suggest the solution for this issue
-
Frank van Puffelen over 8 yearsIf you do
console.log(new Date(snapshot.val()))
, you'll see the date as a string.var date = new Date(snapshot.val())
will give you the date in an object that you can call useful functions on: developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/… -
Matt Jensen over 7 yearsFirebase.ServerValue.TIMESTAMP is a Firebase constant value not a UNIX timestamp.
-
Ayaz Ahmad Tarar over 7 yearsbut it use the format like 1486106680368 how can i convert it into date and time
-
Tope about 7 yearsthe return value after setting it in the database is a UNIX timestamp but the actual command is not, so while yes at some point they resolve to the same thing and can be thusly converted, one must be careful to not try an duse the Firebase timestamp value the same way they would the getTime function in an operation.
-
Rahul Singh almost 7 yearsthe question is for js not java
-
William Wu almost 7 years@AyazAhmadTarar if you are using angular, can simply use their built in pipes such as 'Date', or use angular2-moment to easily convert the timestamp
-
Tiago Gouvêa almost 7 years@WilliamWu but it will use the CLIENT timestamp.. not? The firebase ServerValue.TIMESTAMP ensure that it's the "real" time from server.
-
Rahul Bagal over 5 yearsI am trying to use this solution however I get the following error - ReferenceError: firebase is not defined. I am using js
-
Kyle Finley over 5 yearsRahul, it sounds like you don't have the firebase library installed or loaded. Are you using the web version or the Node JS version? firebase.google.com/docs/firestore/quickstart
-
kai_onthereal almost 5 yearsI found that by adding a new field name 'createdAt' to a 'timestamp' value, it automatically creates a timestamp for all documents
-
Himanshuman over 3 yearsadd explanation
-
Chukwu3meka over 3 yearsI think this is what the user us asking
-
Aydın Candan over 3 yearssee usage section:-)
-
Suyash Vashishtha almost 3 yearsTotally works and returns date in - Day (alphabetic ) month (alphabetic ) date (numerical) year (numerical) time (hh:mm) and Time zone (GMT+ ..). Thanks
-
Rodius about 2 yearsWhy do you multiply seconds * 1000? Thank you
-
vineet about 2 years
new Date(milliseconds)
the function accepts ms, to convert seconds to ms we multiply by 1000 w3schools.com/js/…