firebaseAuth.getCurrentUser() return null DisplayName
Solution 1
This is a tricky one since it is not so clear in the documentation...
Check the getProviderData()
as defined here: https://firebase.google.com/docs/reference/android/com/google/firebase/auth/FirebaseUser#public-method-summary
You can iterate that List and it will have all the providers associated with that account, included a provider with the providerId = "google.com" with a display Name = YOUR_GOOGLE_USERNAME
let me know if you cannot make it work
Solution 2
Just to add to Ymmanuel's answer (Thank you!) with some example code for anyone else looking for a quick copy and paste:
FirebaseUser user = firebaseAuth.getCurrentUser();
if (user != null) {
// User is signed in
String displayName = user.getDisplayName();
Uri profileUri = user.getPhotoUrl();
// If the above were null, iterate the provider data
// and set with the first non null data
for (UserInfo userInfo : user.getProviderData()) {
if (displayName == null && userInfo.getDisplayName() != null) {
displayName = userInfo.getDisplayName();
}
if (profileUri == null && userInfo.getPhotoUrl() != null) {
profileUri = userInfo.getPhotoUrl();
}
}
accountNameTextView.setText(displayName);
emailTextView.setText(user.getEmail());
if (profileUri != null) {
Glide.with(this)
.load(profileUri)
.fitCenter()
.into(userProfilePicture);
}
}
The above will try to use the first display name and photo url from the providers if it wasn't initially found in the User object.
Bonus: Using glide for images: https://github.com/bumptech/glide .
Solution 3
Edmund Johnson is right. This issue was introduced in Firebase Auth 9.8.0. A workaround includes downgrading to 9.6.1 or forcing 're-login' as the info is populated after the user logs out and logs back in. The problem is described in the Firebase issue
It has been reported as a bug to Firebase by one of the Firebase UI contributors - Alex Saveau.
Solution 4
I had the same issue, I solved it by signing out the user and resigning them back in.
Call FirebaseAuth.getInstance().signOut();
to sign them out, then try again.
As I've discovered, this issue is common with using email/password authentication and Social login (Facebook in my case) at the same time.
Solution 5
I found a solution for this problem, in the Firebase documentation! The solution is to update the user profile using the: UserProfileChangeRequest:
UserProfileChangeRequest profileUpdates = new UserProfileChangeRequest.Builder()
.setDisplayName(mUser.getName())
.setPhotoUri(Uri.parse("https://example.com/mario-h-user/profile.jpg"))
.build();
firebaseUser.updateProfile(profileUpdates)
.addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull Task<Void> task) {
if (task.isSuccessful()) {
Log.d(TAG, "User profile updated.");
}
}
});
The variable mUser is already filled with the content from the fields. I used this piece of code inside the FirebaseAuth.AuthStateListener() -> onAuthStateChanged()
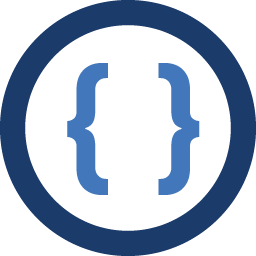
Admin
Updated on June 26, 2022Comments
-
Admin about 2 years
When I signIn with my google account and get the name with the getDisplayName(), my name appear correctly, but in the AuthStateListener doesn't.
here part of my code:
private void handleSignInResult(GoogleSignInResult result) { Alert.dismissProgress(); if (result.isSuccess()) { GoogleSignInAccount acct = result.getSignInAccount(); if(acct != null) { Log.i("NAME", acct.getDisplayName()); <-- RETURN MY NAME CORRECTLY credential = GoogleAuthProvider.getCredential(acct.getIdToken(), null); fuser.linkWithCredential(credential).addOnCompleteListener(authResult); } else { //ERROR } } else { //ERROR } }
But in my AuthStateListener
@Override public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) { FirebaseUser nuser = firebaseAuth.getCurrentUser(); if (nuser != null) { Log.i("NAME", nuser.getDisplayName()); <--- RETURN NULL } }
Somebody know why this can happen?
-
Admin about 8 yearsThanks! getProviderData() return me 2 users informations, so I can select the google.com info, but still weird that if I link the new user it should show me just the new user and not both... but still works ;)
-
Ymmanuel about 8 yearsYea i've noticed a couple of firebase weird stuff.....like for example in iOS the anonymous key returns anonymous = true with custom authentication and with anonymous authentication while in android custom = false and anonymous = true