Firestore-Flutter-GetX: How to get document id to update a record in Firestore
853
static updateProfile(ProfileModel profilemodel, documentId ) {
firebaseFirestore
.collection('users')
.doc(auth.currentUser!.uid)
.collection('profile')
.get()
.then((QuerySnapshot querySnapshot) {
final docId = queryShapchot.docs.first.id;
firebaseFirestore
.collection('users')
.doc(auth.currentUser!.uid)
.collection('profile')
.doc(docId)
.update({
// Add data here
})
});
}
This should do the trick for you.
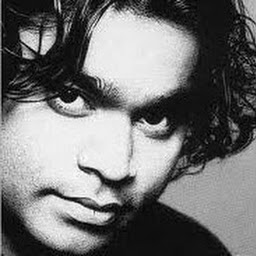
Author by
sandeep rana
Updated on January 04, 2023Comments
-
sandeep rana over 1 year
I am very new to Dart, and coding in general. I have produced this code after watching tutorials on YouTube. For the most part, I have been able to troubleshoot most of my problems on my own, yet I cannot figure out my most recent errors. I was successful to create record in firestorm but I am unable to update it because I am not able to get Doc ID.
Here is my code:-
import 'package:flutter/material.dart'; import 'package:lms_definer/controllers/profileController.dart'; import 'package:lms_definer/controllers/textController.dart'; import 'package:get/get.dart'; import 'package:lms_definer/model/profileModel.dart'; import 'package:lms_definer/constants/constants.dart'; import 'package:cloud_firestore/cloud_firestore.dart'; import '../helper/firestore_db.dart'; class EditProfile extends StatelessWidget { const EditProfile({Key? key}) : super(key: key); @override Widget build(BuildContext context) { var _textController = ProfileEdit(); return Scaffold( body: GetX<ProfileController>( init: Get.put<ProfileController>(ProfileController()), builder: (ProfileController profileController) { return Container( child: ListView.builder( itemCount: profileController.profiles.length, itemBuilder: (BuildContext context, int i) { final _profileModel = profileController.profiles[i]; setTextEditControllerValue(_textController, _profileModel); return SafeArea( child: Container( padding: EdgeInsets.all(20), child: Form( child: Column( children: [ TextFormField( decoration: const InputDecoration( labelText: 'First Name', border: OutlineInputBorder( borderSide: BorderSide()), ), controller: _textController.fNameController ), SizedBox( height: 10, ), TextFormField( decoration: const InputDecoration( labelText: 'First Name', border: OutlineInputBorder( borderSide: BorderSide()), ), controller: _textController.lNameController, ), SizedBox( height: 10, ), TextFormField( decoration: const InputDecoration( labelText: 'Address', border: OutlineInputBorder( borderSide: BorderSide()), ), controller: _textController.adressController, ), SizedBox( height: 10, ), TextFormField( decoration: const InputDecoration( labelText: 'Phone Numbaer', border: OutlineInputBorder( borderSide: BorderSide()), ), controller: _textController.phoneController, ), SizedBox( height: 10, ), TextFormField( decoration: const InputDecoration( labelText: 'School Name', border: OutlineInputBorder( borderSide: BorderSide()), ), controller: _textController.sclNameController, ), SizedBox( height: 10, ), TextFormField( decoration: const InputDecoration( labelText: 'Student Class', border: OutlineInputBorder( borderSide: BorderSide()), ), controller: _textController.stdClassController, ), SizedBox( height: 10, ), ElevatedButton(onPressed: () async { ProfileModel profileModel = profileModelVal(_textController); //await FirestoreDb.updateProfile(profileModel, documentId); }, child: Text('Update')) ], ), ), ), ); }), ); }, )); } ProfileModel profileModelVal(ProfileEdit _textController) { final profileModel = ProfileModel( firstName: _textController.fNameController.text.trim(), lastName: _textController.lNameController.text.trim(), parentName: _textController .fatherNameController.text .trim(), phoneNumber: _textController.phoneController.text.trim(), address: _textController.adressController.text.trim(), schoolName: _textController.sclNameController.text.trim(), stdClass: _textController.stdClassController.text .trim()); return profileModel; } void setTextEditControllerValue(ProfileEdit _textController, ProfileModel _profileModel) { _textController.fNameController.value = TextEditingValue(text: _profileModel.firstName); _textController.lNameController.value = TextEditingValue(text: _profileModel.lastName); _textController.fatherNameController.value = TextEditingValue(text: _profileModel.parentName); _textController.adressController.value = TextEditingValue(text: _profileModel.address); _textController.phoneController.value = TextEditingValue(text: _profileModel.phoneNumber); _textController.sclNameController.value = TextEditingValue(text: _profileModel.schoolName); _textController.stdClassController.value = TextEditingValue(text: _profileModel.stdClass); } void clearTextController(ProfileEdit _textController) { _textController.fNameController.clear(); _textController.lNameController.clear(); _textController.fatherNameController.clear(); _textController.adressController.clear(); _textController.phoneController.clear(); _textController.sclNameController.clear(); _textController.stdClassController.clear(); } }
MY Database file:-
import 'package:cloud_firestore/cloud_firestore.dart'; import 'package:flutter/cupertino.dart'; import 'package:lms_definer/constants/constants.dart'; import 'package:lms_definer/model/profileModel.dart'; class FirestoreDb { static createProfile(ProfileModel profilemodel) async { await firebaseFirestore.collection('users').doc(auth.currentUser!.uid) .collection('profile') .add({ 'firstName': profilemodel.firstName, 'lastName': profilemodel.lastName, 'parentName': profilemodel.parentName, 'phoneNumber': profilemodel.phoneNumber, 'address': profilemodel.address, 'schoolName': profilemodel.schoolName, 'stdClass': profilemodel.stdClass, 'createdOn': Timestamp.now(), 'isDone': true }); } static Stream<List<ProfileModel>> profileStream() { return firebaseFirestore .collection('users') .doc(auth.currentUser!.uid) .collection('profile') .snapshots() .map((QuerySnapshot query) { List<ProfileModel> profiles = []; for (var profile in query.docs) { final profileModel = ProfileModel.fromDocmentSnapshot(documentSnapshot: profile); profiles.add(profileModel); } return profiles; }); } static updateProfile(ProfileModel profilemodel, documentId ) { firebaseFirestore .collection('users') .doc(auth.currentUser!.uid) .collection('profile') .doc(documentId) .update( { 'firstName': profilemodel.firstName, 'lastName': profilemodel.lastName, 'parentName': profilemodel.parentName, 'phoneNumber': profilemodel.phoneNumber, 'address': profilemodel.address, 'schoolName': profilemodel.schoolName, 'stdClass': profilemodel.stdClass, 'createdOn': Timestamp.now(), } ); } static deleteProfile(String documentID) { firebaseFirestore.collection('users').doc(auth.currentUser!.uid).collection( 'profile').doc(documentID).delete(); }}
I need doc Id to update the file. Please help. Thank you
-
sandeep rana about 2 yearsThe profile data is in users collection then uid then profile collection then doc id then comes data.
-
Advait about 2 yearsIf you already have the uid and the issue is getting the doc id in the profile collection.. then you can just pull all the docs in the profile collection and use the doc with index 0. Since each user should ideally have only one profile.
-
sandeep rana about 2 yearsi have added database screen shot. can you help with code?
-
Advait about 2 yearsAdded another answer with the code.
-
sandeep rana about 2 yearsi am getting this error:- Try correcting the name to the name of an existing method, or defining a method named 'update'. querySnapshot.docs[0]!.update({
-
Advait about 2 yearschanged the code to rectify a mistake, please try again to see if this one works.