Flask - Bad Request The browser (or proxy) sent a request that this server could not understand
121,331
The error there is resulting from a BadRequestKeyError
because of access to a key that doesn't exist in request.form
.
ipdb> request.form['u_img']
*** BadRequestKeyError: 400 Bad Request: The browser (or proxy) sent a request that this server could not understand.
Uploaded files are keyed under request.files
and not request.form
dictionary. Also, you need to lose the loop because the value keyed under u_img
is an instance of FileStorage
and not iterable.
@app.route('/', methods=['GET', 'POST'])
def index():
target = os.path.join(app_root, 'static/img/')
if not os.path.isdir(target):
os.makedirs(target)
if request.method == 'POST':
...
file = request.files['u_img']
file_name = file.filename or ''
destination = '/'.join([target, file_name])
file.save(destination)
...
return render_template('index.html')
Related videos on Youtube
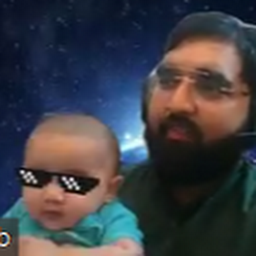
Author by
Roxas Zohbi
Updated on July 17, 2022Comments
-
Roxas Zohbi almost 2 years
I'm trying to do a file upload & enter data task into my MongoDB using flask but I had this error when I filled my form & upload the image:
Bad Request The browser (or proxy) sent a request that this server could not understand.
my HTML code
<form class="form-check form-control" method="post" enctype="multipart/form-data" action="{{ url_for('index') }}"> <label>Full Name*</label></td> <input name="u_name" type="text" class="text-info my-input" required="required" /> <label>Email*</label> <input name="u_email" type="email" class="text-info my-input" required="required" /> <label>Password*</label> <input name="u_pass" type="password" class="text-info my-input" required="required" /> <label>Your Image*</label> <input name="u_img" type="file" class="text-info" required="required" /></td> <input name="btn_submit" type="submit" class="btn-info" /> </form>
& my python code:
from flask import Flask, render_template, request, url_for from flask_pymongo import PyMongo import os app = Flask(__name__) app.config['MONGO_DBNAME'] = 'flask_assignment' app.config['MONGO_URI'] = 'mongodb://<user>:<pass>@<host>:<port>/<database>' mongo = PyMongo(app) app_root = os.path.dirname(os.path.abspath(__file__)) @app.route('/', methods=['GET', 'POST']) def index(): target = os.path.join(app_root, 'static/img/') if not os.path.isdir(target): os.mkdir(target) if request.method == 'POST': name = request.form['u_name'] password = request.form['u_pass'] email = request.form['u_email'] file_name = '' for file in request.form['u_img']: file_name = file.filename destination = '/'.join([target, file_name]) file.save(destination) mongo.db.employee_entry.insert({'name': name, 'password': password, 'email': email, 'img_name': file_name}) return render_template('index.html') else: return render_template('index.html') app.run(debug=True)
-
Rafael Paz about 5 yearsthanks mate your answered solved my problem. I had my html input text with NO property "name". After place that everything worked just fine. Here's the working code: <input class="form-control" id="userId" name="userId" placeholder="User Id" type="text" />
-
learner about 5 yearsIn my case, value of name property of input tag was different in HTML and the key I was trying to access inside f = request.files['file'] was different. Changed both to "file" and it worked.