Flask Jinja2 - Parse JSON
Solution 1
I think I got to the end of it.
I had to json_dumps
the object at first, and then to json.loads
the new object.
r = json.dumps(json_onject)
loaded_r = json.loads(r)
return render_template("report.html", items=loaded_r)
And now I can use any value from the JSON object.
Solution 2
I have a slightly modified example that worked really well for me:
app.py:
link_chart_data = {
"type":
"LinkChart",
"items": [{
"type": "node",
"id": "ac1",
"u": "static/icons/bank.png",
"t": "45081063"
}]
}
@app.route('/link_chart')
def covid():
return render_template("link_chart/index.html", flask_data=link_chart_data)
Then in templates/link_chart/index.html:
<script>
const parsedData = JSON.parse('{{ flask_data|tojson }}');
const unparsedData = '{{ flask_data }}'
console.log('parsed', parsedData)
console.log('unparsed', unparsedData)
</script>
Which yields:
parsed,
items: Array(1)
0:
id: "ac1"
t: "45081063"
type: "node"
u: "static/icons/bank.png"
__proto__: Object
length: 1
__proto__: Array(0)
type: "LinkChart"
^^This is what I want. a new JSON object
and:
unparsed {'type': 'LinkChart', 'items': [{'type': 'node', 'id': 'ac1', 'u': 'static/icons/bank.png', 't': '45081063'}]}
^^Which I really don't want.
The |tojson
jinja template un-asciis the variable so that it gets passed as the same format it came from python (flask). the JSON.parse()
javascript function is what converts it from a string to a JSON object in javascript.
I hope this helps.
Solution 3
Hey so the json you have is an array not an object so you have to take that into consideration and access the object you want first and then access the attribute.
<div id="name">{{ items[0]['name'] }}</div>
or
In this case x
becomes the object and you can use it like so:
{% for x in items %}
<div id="name">{{ x['name'] }}</div>
{% endfor %}
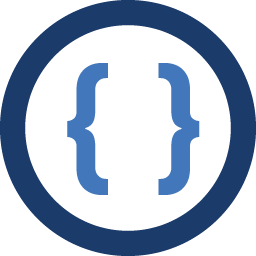
Admin
Updated on April 30, 2020Comments
-
Admin about 4 years
I'm trying do display some JSON results in a Jinja2 HTML Template, but it returns either an empty page, or every character of the JSON on a new row, or every character with spacing.
The object that I'm sending to Jinja2 Template looks like this:
[ { "name": "John", "lastname": "Smith", "age": 22, "likes": [ {"fruits": ["bananas", "oranges"] }, {"sweets": ["chocolate"] } ] } ]
To send the JSON object to the Jinja2 template I use:
render_template("report.html", items=items)
On the Jinja2 Template HTML if I use
{{ items }}
it displays the full object as text. If I'm trying to get the{{ items['name'] }}
it will not display anything on the page, the page will be completely empty.I have also tried
{% for x in items %} {{ x }} {% endfor %}
and it only displays the same "text" but with spacing:
[ { " n a m e " : "J o h n " [...]
In the HTML file, I'm trying to do something like:
<div id="name">{{ items['name'] }}</div>
How can I display the objects within the JSON object I'm passing to Jinja2?
-
Shawn K almost 6 yearsWhen you say JSON... you mean python object? Jinja2 renders python object to templates.
-
Admin almost 6 yearsdictionary, yes...
-
-
Admin almost 6 yearsThanks for your reply.. However, if I'm making an object ` items = dict() items['name'] = "John" return render_template("report.html", items=items)` and try to print items['name'] it still returns an empty page.
-
Rodrigo E. Principe about 3 yearsthank you @Sharpie, it worked like a charm =)