Flutter app crashes on firebase phone auth in iOS platform
2,577
i Solved the Problem, thank you so much @DevarshRanpura for Helping me,
1) replace
signIn(){
final AuthCredential credential = PhoneAuthProvider.getCredential(
verificationId: verificationId,
smsCode: smsCode);
FirebaseAuth.instance.signInWithCredential(credential).then((user){
Navigator.of(context).pushReplacementNamed('/homepage');
}).catchError((e){
print('Auth Credential Error : $e');
});
}
to this
signIn() async {
final AuthCredential credential = PhoneAuthProvider.getCredential(
verificationId: verificationId,
smsCode: smsCode,
);
final AuthResult authResult = await _auth.signInWithCredential(credential);
final FirebaseUser user = authResult.user;
print('User Id : ' + user.uid);
}
also Define _auth on your dart file
final FirebaseAuth _auth = FirebaseAuth.instance;
2) adding reverse client id in to your project
- open your project in xcode
- double click on your project name
- go to the info tab
- In URL Types->URL schemes add the reverse client Id
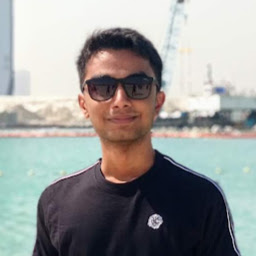
Author by
dhruvin prajapati
Updated on December 18, 2022Comments
-
dhruvin prajapati 5 months
I have implemented Firebase Phone auth in my project,
On android side everything works fine, but for iOS side, when i try to send verification code from my end, app crashes and lost connection.
I have submitted my code below.
main.dart
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return new MaterialApp( home: new MyHomePage(), routes: <String, WidgetBuilder> { '/homepage': (BuildContext context) => MyApp(), '/landingpage': (BuildContext context) => MyHomePage() } ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => new _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { String phoneNo; String smsCode; String verificationId; Future<void> verifyPhone() async { print("main"); final PhoneCodeAutoRetrievalTimeout autoRetrieve = (String verId) { print("varification id"); this.verificationId = verId; }; final PhoneCodeSent smsCodeSent = (String verId, [int forceCodeResend]) { print("send code dilog"); this.verificationId = verId; smsCodeDialog(context).then((value) { print('Signed in'); }); }; final PhoneVerificationCompleted verifiedSuccess = (AuthCredential user){ print('Phone Verification Completed'); }; final PhoneVerificationFailed veriFailed = (AuthException exception) { print('${exception.message}'); }; await FirebaseAuth.instance.verifyPhoneNumber( phoneNumber: "+919700000000", codeAutoRetrievalTimeout: autoRetrieve, codeSent: smsCodeSent, timeout: const Duration(seconds: 5), verificationCompleted: verifiedSuccess, verificationFailed: veriFailed); } Future<bool> smsCodeDialog(BuildContext context) { return showDialog( context: context, barrierDismissible: false, builder: (BuildContext context) { return new AlertDialog( title: Text('Enter sms Code'), content: TextField( onChanged: (value) { this.smsCode = value; }, ), contentPadding: EdgeInsets.all(10.0), actions: <Widget>[ new FlatButton( child: Text('Done'), onPressed: () { FirebaseAuth.instance.currentUser().then((user) { if (user != null) { Navigator.of(context).pop(); Navigator.of(context).pushReplacementNamed('/homepage'); } else { Navigator.of(context).pop(); signIn(); } }); }, ) ], ); }); } signIn(){ final AuthCredential credential = PhoneAuthProvider.getCredential( verificationId: verificationId, smsCode: smsCode); FirebaseAuth.instance.signInWithCredential(credential).then((user){ Navigator.of(context).pushReplacementNamed('/homepage'); }).catchError((e){ print('Auth Credential Error : $e'); }); } @override Widget build(BuildContext context) { return new Scaffold( appBar: new AppBar( title: new Text('PhoneAuth'), ), body: new Center( child: Container( padding: EdgeInsets.all(25.0), child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ TextField( decoration: InputDecoration(hintText: 'Enter Phone number'), onChanged: (value) { this.phoneNo = value; }, ), SizedBox(height: 10.0), RaisedButton( onPressed: verifyPhone, child: Text('Verify'), textColor: Colors.white, elevation: 7.0, color: Colors.blue) ], )), ), ); } }
this is error while submit the button
Launching lib/main.dart on Dhruvin’s iPhone in debug mode... Automatically signing iOS for device deployment using specified development team in Xcode project: ****** Running Xcode build... Xcode build done. 99.4s Installing and launching... Syncing files to device Dhruvin’s iPhone... Lost connection to device.
However i am not able to see stacktrace or log as app lost connection from debugger.
-
Valentin Seehausen almost 3 years"In URL Types->URL schemes add the reverse client Id" -> can you explain this, please?
-
Valentin Seehausen almost 3 yearsHere is a good explanation: stackoverflow.com/questions/61514076/…
-
dhruvin prajapati almost 3 yearsthank you @ValentinSeehausen for more visual explaning the problem, now more devlopers can easy to solve this issue.