flutter await result always null
1,754
The problem with your function is that it's not returning a Future, but the Address object instead. I would rewrite your function like this to just return the Address object
Future<Address> readAddress() async {
try{
database = FirebaseDatabase(app: app);
DataSnapshot snapshot = await database
.reference()
.child(table_name)
.child(uid)
.once();
return Address().map(snapshot.value);
}catch(e) {
print(e);
return(e);
}
}
With this, your function call can be just this:
Address address = readAddress();
Simple, isn't it? I have taken care all of the error handling inside the function.
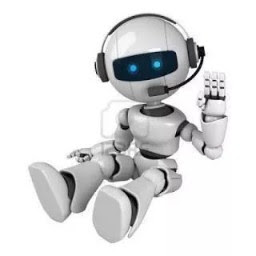
Author by
Midhilaj
Updated on December 08, 2022Comments
-
Midhilaj over 1 year
This is my function
Future<Address> readAddress() async { database = FirebaseDatabase(app: app); await database .reference() .child(table_name) .child(uid) .once() .then((DataSnapshot snapshot) { print("address_start"); if (snapshot.value == null) { print("address_start_value_null"); return null; } else { print("address_start_value_not_null"); print(snapshot.value); Address a = Address().map(snapshot.value); return a; // return a; } }).catchError((onError) { print(onError); return onError; }); }
This is my function call
readAddress().then((address) { if (address != null) { print("address read seucssfully " + address.firstname); } else { print( "address read faield result is null $address"); // + address.toString()); } }).catchError((onError) { print("error on read address"); });
But here always it returns null.
What is wrong here?message from readAddress() function
[dart] This function has a return type of 'Future', but doesn't end with a return statement. [missing_return]
I don't know to explain more StackOverflow showing this error message when in try to post this question "t looks like your post is mostly code; please add some more details. "-
Noodles over 5 yearsIs
print("address_start_value_null")
called? -
Midhilaj over 5 yearsno, it is not called
-
Midhilaj over 5 yearsit contain value i checked
-
Midhilaj over 5 yearsaddress_start_value_not_null is called
-
-
Vas over 5 yearsI don't see how this would work as functions marked with
async
are supposed to return aFuture
-
dshukertjr over 5 years@Vas You need the function to be async, because it contains await statement.
-
Vas over 5 yearsRight, but then the function return type has to be
Future<Address>