Flutter BLoC: Are Cubits better then BLoC?
Solution 1
BLoC
The advantage of having events instead of direct method calls is that you can debounce/throttle, buffer the stream before doing your logic.
In other words, you can use special methods applicable for events logic.
Cubit
If you start with Cubit for a new project, the idea why it exists is that later you will have the ability to migrate from Cubit to BLoC.
This means that if at the beginning of a project you think that BLoC is too much overhead and you need simpler state management (without events, boilerplate, etc.) you can choose cubit and migrate to BLoC with fewer efforts, than if you selected a different state management solution like MobX or Riverpod.
So with Cubit, you first implement the state and functions. Later if you decide to switch to BLoC you add events and EventHandler.
More you can read here (official docs): Cubit vs BLoC
Solution 2
There's a good overview of Cubit vs Bloc in the official documentation.
In short, Cubit's advantage is simplicity, while Bloc provides better traceability and advanced ReactiveX operations.
In our projects, we use both Cubit for simpler cases, and Bloc if the logic is more complicated and some "limitations" actually become useful:
- You can emit a new state only as a reaction to an event, so the implementation is more straightforward (but more verbose as well).
- All the events are processed one by one. Again, it makes implementation more reliable and easier to maintain.
Also, that can be a matter of personal preference, but I like Bloc for its close mapping to the FSM pattern. In most cases, the application state can be nicely represented as a state machine. It's easier to discuss the implementation even with a whiteboard, as you can just show the scheme with a number of states and events changing that state.
If you're happy with Cubit, then you probably don't need Bloc. After all, the main goal is to make the architecture easy to understand and to maintain.
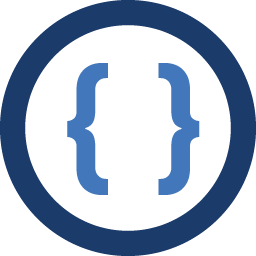
Admin
Updated on December 15, 2022Comments
-
Admin over 1 year
I'm working with Flutter quite a long time and have a bunch of released products. I never really liked BLoC and preferred to use Provider or later Riverpod.
I just don't understand that event concept. Why do we still need it? And i'm confused because of it's actual popularity... BLoC has Cubit subclass that seems to be more simple to use, but everyone just keep telling: "Cubit is simpler, but not so functional". But what are limitations?
I even think that Cubits are MORE USEFUL and MORE SIMPLE at the same time:
- With Cubit you just call it's method with params. You still can listen its state AND get the method return value if needed too.
- You don't need extra coding implementing these Event Types.
- You don't need extra extra coding implementing how bloc will handle every single event type. METHODS DO THAT JUST FINE.
example: User taps some product's "Add to cart" button.
Cubit:
cartCubit.addProduct(productId);
BLoC:
cartBloc.addEvent(UserAddsProductEvent(productId));
inside them:
Cubit:
void addProduct(String productId) async { //some validation... if(...){...} final result = await cartRepo.addProduct(id); if(result == ...) { state = someState; //.... }
BloC:
void addEvent(CartEvent event) { if (event is UserAddsProductEvent) { _addProduct(event.productId) } else if (event is....) { //..... } } void _addProduct(String productId) async { //some validation... if(...){...} final result = await cartRepo.addProduct(id); if(result == ...) { state = someState; //.... }
What is the point?