Flutter/Dart How to adjust Modalbottomsheet animation speed?
If you are using a StatefulWidget add with TickerProviderStateMixin
and create an AnimationController
with BottomSheet.createAnimationController(this)
. You can then set the duration
on the AnimationController. In this example I've set the duration to 3 seconds.
Make sure you dispose the AnimationController in void dispose ()
class MyModalBottomButton extends StatefulWidget {
@override
_MyModalBottomButtonState createState() => _MyModalBottomButtonState();
}
class _MyModalBottomButtonState extends State<MyModalBottomButton>
with TickerProviderStateMixin {
AnimationController controller;
@override
initState() {
super.initState();
controller =
BottomSheet.createAnimationController(this);
controller.duration = Duration(seconds: 3);
}
@override
void dispose() {
controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return TextButton(
child: Text("Show bottom sheet"),
onPressed: () => showModalBottomSheet(
context: context,
transitionAnimationController: controller,
builder: (context) {
return Container(
child: Text("Your bottom sheet"),
);
},
),
);
}
}
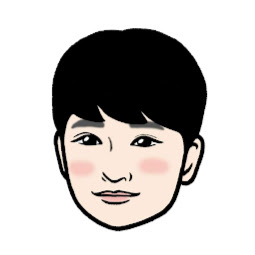
Hajime
Updated on December 28, 2022Comments
-
Hajime 5 months
I read this reference
https://api.flutter.dev/flutter/material/showModalBottomSheet.html
it says "transitionAnimationController parameters can be passed in to customize the appearance and behavior of modal bottom sheets. The transitionAnimationController controls the bottom sheet's entrance and exit animations if provided."
but, I couldn't find any reference of transitionAnimationController,
so my question is, How can I adjust ModalBottomSheet Animation(entrance and exit speed that I want to adjust) with transitionAnimationController?
thank you.
-
Hajime about 2 yearsthank you so much, it solved my problem. you are the best!
-
Lidor Eliyahu Shelef almost 2 yearsit solves the problem but after you close it it wont let you reopen it for some assertion error
-
Angelica almost 2 yearsException caught by gesture ══════════════════════════════════════════════ The following assertion was thrown while handling a gesture: AnimationController.forward() called after AnimationController.dispose() AnimationController methods should not be used after calling dispose. 'package:flutter/src/animation/animation_controller.dart': Failed assertion: line 455 pos 7: '_ticker != null'
-
lokesh-coder almost 2 years@Angelica I got the same issue. temporary solution is to add listener again in onComplete
showModalBottomSheet().whenComplete(() {controller =BottomSheet.createAnimationController(this);});
-
makri aymen abderraouf over 1 yearhow can I change the closing animation of bottomSheet!
-
Moh. Absar Rahman over 1 yearFor changing the closing animation, you have to use reverse duration
controller = BottomSheet.createAnimationController(this); controller.duration = Duration(seconds: 3); controller.reverseDuration = Duration(seconds: 3);