flutter dart JsonSerializable with inherited class
4,095
Solution 1
this one I have solved by passing the parameters to super in verify class constructor like this
@JsonSerializable()
class VerifyResponse extends Response {
Data data;
VerifyResponse({
this.data,
String responseCode,
String responseMessage,
}) : super(responseCode: responseCode, responseMessage: responseMessage);
factory VerifyResponse.fromJson(Map<String, dynamic> json) =>
_$VerifyResponseFromJson(json);
Map<String, dynamic> toJson() => _$VerifyResponseToJson(this);
}
and for the response class it remains the same
@JsonSerializable()
class Response {
final String responseCode;
final String responseMessage;
Response({this.responseCode, this.responseMessage});
factory Response.fromJson(Map<String, dynamic> json) =>
_$ResponseFromJson(json);
}
it's a bit annoying but it's what it's.
Solution 2
You should remove 'final' keyword from Response Class
@JsonSerializable(nullable: true)
class Response {
String responseCode;
String responseMessage;
String errorLog;
Response({this.errorLog, this.responseCode, this.responseMessage});
factory Response.fromJson(Map<String, dynamic> json) =>
_$ResponseFromJson(json);
}
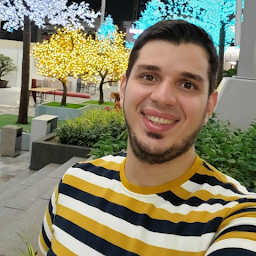
Author by
Baraa Aljabban
Updated on December 21, 2022Comments
-
Baraa Aljabban over 1 year
I have the following two classes where one is extending from the other like this :
@JsonSerializable(nullable: true) class Response { final String responseCode; final String responseMessage; final String errorLog; Response({this.errorLog, this.responseCode, this.responseMessage}); factory Response.fromJson(Map<String, dynamic> json) => _$ResponseFromJson(json); }
.........................................................
@JsonSerializable(nullable: false) class Verify extends Response { Data data; Verify({ this.data, }); factory Verify.fromJson(Map<String, dynamic> json) => _$VerifyFromJson(json); Map<String, dynamic> toJson() => _$VerifyToJson(this); }
and whenever I'm trying to read response class properties from Verify class, it's always null.
so please how to achieve this?