Flutter - Draw a triangle in bottom right corner using customPainter
Solution 1
You can easily add another path object and draw it again with canvas.
@override
void paint(Canvas canvas, Size size) {
final paintOrange = Paint()..color = Colors.orange;
final paintGreen = Paint()..color = Colors.green;
final pathOrange = Path();
pathOrange.lineTo(0, size.height / 3);
pathOrange.lineTo(size.width, 0);
pathOrange.close();
final pathGreen = Path();
pathGreen.moveTo(size.width, size.height);
pathGreen.lineTo(0, size.height);
pathGreen.lineTo(size.width, size.height / 1.5);
pathGreen.close();
canvas.drawPath(pathOrange, paintOrange);
canvas.drawPath(pathGreen, paintGreen);
}
Solution 2
First, you have to do a little math:
After you know where you need your points to be, you can draw your lines.
final pathOrange = Path();
// Create your line from the top left down to the bottom of the orange
pathOrange.lineTo(0, size.height * .3387);
// Create your line to the bottom right of orange
pathOrange.lineTo(size.width, size.height * .162);
// Create your line to the top right corner
pathOrange.lineTo(size.width, 0); // 0 on the Y axis (top)
// Close your line to where you started (0,0)
pathOrange.close();
// Draw your path
canvas.drawPath(pathOrange, _paintOrange);
Now repeat those steps with one addition: moveTo
. By default your path will start at the origin (0, 0) top-left. However, you want it to start at a new location.
final pathGreen = Path();
// Create your line from the top left down to the bottom of the orange
pathGreen.moveTo(0, size.height * .978); // (100 - 2.8) / 100
// Create your line to the bottom left
pathGreen.lineTo(0, size.height);
// Create your line to the bottom right
pathGreen.lineTo(size.width, size.height);
// Create your line to top right of green
pathGreen.lineTo(size.width, size.height * .6538); // (100 - 34.62) / 100
// Close your line to where you started
pathGreen.close();
// Draw your path
canvas.drawPath(pathGreen, _paintGreen);
Solution 3
Maybe you can try something like this?
final pathBlue = Path();
pathBlue.moveTo(size.width, size.height);
pathBlue.lineTo(size.width, (size.height/3)*2);
pathBlue.lineTo(0, size.height);
pathBlue.close();
canvas.drawPath(pathBlue, _paintBlue);
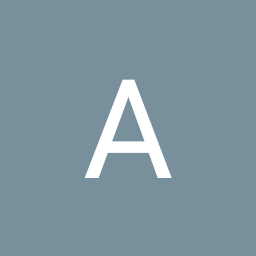
StefanSpeterDev
Web dev @Akabia in Lille, France. Main techs used are Drupal8, php, js, jQuery.
Updated on December 23, 2022Comments
-
StefanSpeterDev over 1 year
I'm trying to draw a triangle in the bottom right of the page.
I've successfully created one in the top left doing like this:void paint(Canvas canvas, Size size) { // top left final pathOrange = Path(); pathOrange.lineTo(0, size.height/3); pathOrange.lineTo(size.width/1.5, 0); pathOrange.close(); canvas.drawPath(pathOrange, _paintOrange); }
But I can't find a way to do the same for the bottom right. I've read that apparently
canvas
is set to0,0
by default, but I don't seem to be able to instantiate it twice, otherwise I would change the initial start usingcanvas.translate
.
I know that bottom-left coordinates are0,size.height
and top-rightsize.width,0
but I just can't get the bottom right one.
That's what the result should be
And here what I've done -
StefanSpeterDev over 3 yearsAwesome answer that works too thank you! I've noticed that instead of
pathGreen.drawPath(pathGreen, _paintGreen);
it should becanvas.drawPath(pathGreen, _paintGreen);
otherwise works like a charm! -
SupposedlySam over 3 yearsThanks for that. I've updated my answer. Also, if you haven't seen this article I highly recommend it: medium.com/flutter-community/…
-
StefanSpeterDev over 3 yearsthanks for article, indeed despite many google research I didn't got on it, looks very complete thx!