Flutter Firebase foreground push notification not showing but background is working
I dont know if it will work or not for you, but here is my code to show notification when app on foreground. I put messageHandler on initState(). LocalNotification
is a custom class, so you can either create a same class, or just put whatever inside showNotification
to onMessage
Future<void> messageHandler() async {
await FirebaseMessaging.instance.setForegroundNotificationPresentationOptions(
alert: true,
badge: true,
sound: true,
);
FirebaseMessaging.onMessage.listen((RemoteMessage event) {
LocalNotification.showNotification(event);
});
}
static Future<void> showNotification(RemoteMessage payload) async {
var android = AndroidInitializationSettings('logo_rs');
var initiallizationSettingsIOS = IOSInitializationSettings();
var initialSetting = new InitializationSettings(android: android, iOS: initiallizationSettingsIOS);
final FlutterLocalNotificationsPlugin flutterLocalNotificationsPlugin = FlutterLocalNotificationsPlugin();
flutterLocalNotificationsPlugin.initialize(initialSetting);
const AndroidNotificationDetails androidDetails = AndroidNotificationDetails(
'default_notification_channel_id',
'Notification',
'All Notification is Here',
importance: Importance.max,
priority: Priority.high,
ticker: 'ticker',
icon: "logo_rs",
playSound: true,
sound: RawResourceAndroidNotificationSound("notification")
);
const iOSDetails = IOSNotificationDetails();
const NotificationDetails platformChannelSpecifics = NotificationDetails(android: androidDetails, iOS: iOSDetails);
await flutterLocalNotificationsPlugin.show(0, payload.notification!.title, payload.notification!.body, platformChannelSpecifics);
}
Add try to put this on your AndroidManifest
<meta-data android:name="com.google.firebase.messaging.default_notification_channel_id"
android:value="@string/default_notification_channel_id" />
String.xml (new file inside res/values)
<?xml version='1.0' encoding='utf-8'?>
<resources>
<string name="default_notification_channel_id" translatable="false">fcm_default_channel</string>
</resources>
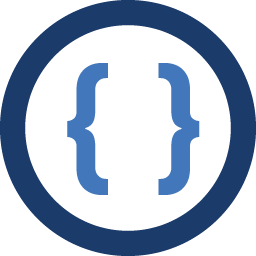
Admin
Updated on January 02, 2023Comments
-
Admin 5 months
It's been few days since I've been trying to solve this problem. I have implemented firebase messaging for push notification, as a result, I am able to receive notification when the app is in background, but not able to receive it when the app is in foreground. I tried every solution possible or on stackoverflow/github but nothing worked so far. The following are the sources which I tried:
Current code =>
NotificationService.dart
:class NotificationService { static final FlutterLocalNotificationsPlugin _flutterLocalNotificationsPlugin = FlutterLocalNotificationsPlugin(); static Future initialize() async { final FlutterLocalNotificationsPlugin flutterLocalNotificationsPlugin = FlutterLocalNotificationsPlugin(); AndroidInitializationSettings androidInitializationSettings = AndroidInitializationSettings('@mipmap/ic_launcher'); InitializationSettings initializationSettings = InitializationSettings(android: androidInitializationSettings); await flutterLocalNotificationsPlugin.initialize( initializationSettings, // onSelectNotification: doSomething(), ); } static Future showNotification(RemoteMessage message) async { AndroidNotificationDetails androidDetails = AndroidNotificationDetails( "default_notification_channel_id", "channel", enableLights: true, enableVibration: true, priority: Priority.high, importance: Importance.max, largeIcon: DrawableResourceAndroidBitmap("ic_launcher"), styleInformation: MediaStyleInformation( htmlFormatContent: true, htmlFormatTitle: true, ), playSound: true, ); await _flutterLocalNotificationsPlugin.show( message.data.hashCode, message.data['title'], message.data['body'], NotificationDetails( android: androidDetails, )); } }
main.dart
:Future<void> _firebaseMessagingBackgroundHandler(RemoteMessage message) async { await Firebase.initializeApp(); print('Handling a background message ${message.messageId}'); print('Notification Message: ${message.data}'); NotificationService.showNotification(message); } void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); FirebaseMessaging.onBackgroundMessage(_firebaseMessagingBackgroundHandler); runApp( MyApp(), ); } class MyApp extends StatefulWidget { const MyApp({Key? key}) : super(key: key); @override State<MyApp> createState() => _MyAppState(); } class _MyAppState extends State<MyApp> { @override void initState() { super.initState(); NotificationService.initialize(); FirebaseMessaging.onMessage.listen((RemoteMessage message) { NotificationService.showNotification(message); }); } @override Widget build(BuildContext context) { return MaterialApp(...)
One more point to add, when the app is in background (the case where I can receive notification), I get the output of the first 2
print
statements i.e.message.messageId
andmessage.data
:I/flutter ( 3363): Handling a background message 0:1637822186109880%5dd28a92f9fd7ecd I/flutter ( 3363): Notification Message: {body: Price is at Bull! Buy Now!, title: Hello Traders!}
But when the app is in foreground (the case where I do not receive notification), I get the following output:
D/FLTFireMsgReceiver( 3363): broadcast received for message
It would really help if someone could help me out, It's been days debugging, finding solution. Thank you!
AndroidManifest.xml
:<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.trading_app"> <uses-permission android:name="android.permission.VIBRATE"/> <uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED"/> <uses-permission android:name="android.permission.INTERNET"/> <application android:label="TradingApp" android:icon="@mipmap/ic_launcher"> <meta-data android:name="com.google.firebase.messaging.default_notification_icon" android:resource="@mipmap/ic_launcher" /> <meta-data android:name="com.google.firebase.messaging.default_notification_channel_id" android:value="@string/default_notification_channel_id" /> <receiver android:name="com.dexterous.flutterlocalnotifications.ScheduledNotificationBootReceiver"> <intent-filter> <action android:name="android.intent.action.BOOT_COMPLETED"></action> </intent-filter> </receiver> <receiver android:name="com.dexterous.flutterlocalnotifications.ScheduledNotificationReceiver" /> <activity android:name=".MainActivity" android:launchMode="singleTop" android:theme="@style/LaunchTheme" android:configChanges="orientation|keyboardHidden|keyboard|screenSize|smallestScreenSize|locale|layoutDirection|fontScale|screenLayout|density|uiMode" android:hardwareAccelerated="true" android:windowSoftInputMode="adjustResize"> <meta-data android:name="io.flutter.embedding.android.NormalTheme" android:resource="@style/NormalTheme" /> <meta-data android:name="io.flutter.embedding.android.SplashScreenDrawable" android:resource="@drawable/launch_background" /> <intent-filter> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity> <meta-data android:name="flutterEmbedding" android:value="2" /> <!-- <meta-data android:name="com.google.firebase.messaging.default_notification_channel_id" android:value="high_importance_channel" /> --> </application> </manifest>
-
Manish about 1 yearany updates on this, i am also facing the same issue?
-
-
Admin over 1 yearHi, thanks for your answer but I don't think so there is anything I missed according to your answer! Still it's not showing and there's no error...
-
Ananda Pramono over 1 yearTry putting this in your AndroidManifest
<meta-data android:name="com.google.firebase.messaging.default_notification_channel_id" android:value="@string/default_notification_channel_id" />
And change your cahnnel ID to 'default_notification_channel_id' -
Admin over 1 yearI did as you told but I'm getting the following error while debugging (build failed):
Element meta-data#com.google.firebase.messaging.default_notification_channel_id at AndroidManifest.xml:61:13-63:55 duplicated with element declared at AndroidManifest.xml:16:9-18:71
. I think this is because of duplicatemeta-data
?. I have edited the question withAndroidManifest.xml
. -
Admin over 1 yearOkay, that was because there was a duplicate
meta-data
(the last one), I commented that and it build successfully but still, I cannot see the foreground notification, background notification working as usual... -
Admin over 1 yearStill, the same output, and how does checking some print statements solve the problem?
-
Peter Obiechina over 1 yearYour problem was that foreground notification was not showing. My code above would confirm that your device received it. Then you know the error is from
showNotification()
. When you say "same output", does your code print "handling a foreground message..."? -
Admin over 1 yearNo, it doesn't print
Handling a foreground message
, it just printsD/FLTFireMsgReceiver( 2809): broadcast received for message
. Okay, I get your point, so what could be wrong inshowNotification
because it is working withbackground
...