Flutter FutureBuilder widget not responding to Future resolution when chaining Futures with await
You can do it this way since you mentioned in the comments that you want to use async/await only:
void initFutures() {
delayed = fordelayed();
delayedAwaited = fordelayedAwaited();
}
Future<int> fordelayed() async {
return await Future.delayed(Duration(seconds: 2), () => 5);
}
Future<int> fordelayedAwaited() async {
int fromdelayed = await fordelayed();
return fromdelayed * 2;
}
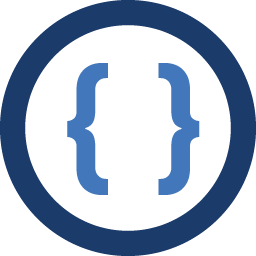
Admin
Updated on December 27, 2022Comments
-
Admin 5 months
I am using a flutter widget's
initState()
method to create two future ints;delayed
which is a simpleFuture.delay
, anddelayedAwaited
, which awaits on thedelayed
variable and doubles its value. Thebuild()
method includes twoFutureBuilder
s, one for each future. See example code below:class FutureTest extends StatefulWidget { @override _FutureTestState createState() => _FutureTestState(); } class _FutureTestState extends State<FutureTest> { Future<int>? delayed; Future<int>? delayedAwaited; @override void initState() { super.initState(); initFutures(); } void initFutures() async { delayed = Future.delayed(Duration(seconds: 2), () => 5); delayedAwaited = Future.value((await delayed)! * 2); } @override Widget build(BuildContext context) { return Column( mainAxisSize: MainAxisSize.min, children: [ FutureBuilder( future: delayed, builder: (context, snapshot) => Text('delayed: ${snapshot.data}'), ), FutureBuilder( future: delayedAwaited, builder: (context, snapshot) => Text('awaited: ${snapshot.data}'), ), ], ); } }
Here is the problem: The UI only updates for
delayed
and notdelayedAwaited
(it remains as null). The UI is updated to reflect the true value when manually hot-reloading, so the future is resolving correctly, but Flutter is just not triggering theFutureBuilder
to rebuild. My expectation would be that the UI updates fordelayAwaited
as well.See the behavior here: https://dartpad.dev/62b272db200ca39ed854be5a7183967d?null_safety=true
For some reason, changing
initFutures()
so it usesFuture.delayed
for both futures fixes the issue, as shown here: https://dartpad.dev/9b386a45428046b193800351a4ade5b1?null_safety=trueIs anyone able to explain why the given code works like this, and what is best practice for achieving what I am trying to do.
-
Admin over 2 yearsI think this is the best solution. You can simplify further by removing
async
frominitFutures()
. I ended up going with the following syntax, for brevity:delayedAwaited = Future(() async => (await delayed)! * 2);
. The pattern is to assign futures without using async/await directly, but use them within the callback functions (named as yours, or anonymous as mine). -
Calvin Gonsalves over 2 yearsI agree, the
async
frominitFutures()
is not needed. I will update it.