Flutter Hot reloading not working in android studio(mac)
Solution 1
Flutter Documentation says "Hot reload loads code changes into the VM and re-builds the widget tree, preserving the app state; it doesn’t rerun main() or initState()"
So, it looks like if the app logic resides outside the main method, then Hot Reloading works. Try to refactor your app like below and then do Restart (green button). Any subsequent changes you make, will be HOT RELOADED when saved.
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: new Scaffold(
appBar: new AppBar(
title: new Text("Demo Project"),
),
body: Center(child: new Text("Hello World!!!")),
),
);
}
}
UPDATE: Hot Reload will not work if you are adding new assets (like images, or medial files). In those cases you will have to restart the App.
Solution 2
Try This
The solution on GitHub Reloaded 0 of NNN libraries in Xms
Android Studio uses IDEA to import files automatically, Like this
import 'file///F:/flutter_project/lib/home_page.dart';
Change it to
import 'package:flutter_project/home_page.dart';
Solution 3
in the terminal, run
flutter doctor -v
flutter upgrade
Also, try File > Invalidate Caches / Restart...
from Android studio menu
Solution 4
Wrap your code with a StatefulWidget
like shown in the code below.
This might be confusing when watching old tutorials because it used to work without it on an earlier Flutter Version.
import 'package:flutter/material.dart';
void main() {
runApp(new MaterialApp(home: MyApp()));
}
class MyApp extends StatefulWidget {
MyApp({Key key}) : super(key: key);
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text("Demo Project"),
),
body: Center(child: new Text("Hello World!!!")),
);
}
}
Solution 5
If I'm not mistaken, hot reload calls the build method of your widget, as the change you made weren't made inside a build method, it didn't change anything in the screen.
Try changing the texting inside the MyWidget Build method and see if it works.
import 'package:flutter/material.dart';
void main() {
runApp(new MaterialApp(
home: new Scaffold(
appBar: new AppBar(
title: new Text("Demo Project"),
),
body: new MyWidget(),
),
));
}
class MyWidget extends StatelessWidget {
const MyWidget({
Key key,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Center(child: new Text("Hello World!!!"));
}
}
Related videos on Youtube
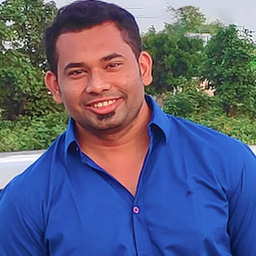
Jitesh Mohite
I am a technology enthusiast who has 7 plus years of working experience in the mobile domain. I want to learn things quickly and dive deep inside it. I always believe in developing logical things that make an impact on end-users. I worked on Android, Flutter, Python, and automation. I am an active member of StackOverflow, GitHub, and a medium community, where I help others to solve their difficulty. Email Id: [email protected] Some of my contributions GitHub: https://github.com/jitsm555 Medium: https://medium.com/flutterworld Youtube Channel: Flutter World I have started this channel to help flutter community Flutter Interview Questions
Updated on March 02, 2022Comments
-
Jitesh Mohite about 2 years
While working on mac machine I found that hot reloading not working in Android Studio. I don't know what's wrong :)
Here is the code which not reloading(I am changing the Hello world text to Flutter Sample text)
import 'package:flutter/material.dart'; void main() { runApp(new MaterialApp( home: new Scaffold( appBar: new AppBar( title: new Text("Demo Project"), ), body: Center(child: new Text("Hello World!!!")), ), )); }
Please see this below video with the link https://www.dropbox.com/s/e7viujcgv8w0mtr/hot_reload.mp4?dl=0
This not stop my development, But I am concerned why it is happening.
-
shb almost 5 yearsThis is weird, it happened to me couple of times too. I closed everything, restarted the project and then it worked fine.
-
ישו אוהב אותך almost 5 yearsMy question here a little out of the topic, did the some problem is happened when you're using IntelliJ?
-
Jitesh Mohite almost 5 yearsI am not using IntelliJ, sorry.
-
neobie about 4 yearsMy hot reload is working in Visual studio code, but not working in Android Studio, any one ?
-
Piyin almost 4 yearsI think the correct answer is Invin's; stackoverflow.com/a/61567821/5503625 You should mark it as accepted
-
-
Luiz França almost 5 yearsI had this problem once and closing android studio and reopening did the trick. @jitsm555.
-
George almost 5 yearsThe video on the question shows the hot reload happening, the flutter message, but nothing happening on the emulator, so it's not a shortcut issue.
-
Simple UX Apps over 4 yearsFile > Invalidate Caches / Restart solved it for me. Thanks.
-
snake_eyes over 4 yearshow to update it? although I applied the command flutter upgrade and got everything is update 2 date.
-
Abdoulaye BARRY over 4 yearsDelete your flutter sdk. Download and extract the new from flutter.io website.
-
Vu Pham almost 4 yearsThis solves my problem. I am working with Flutter version 1.18.0-11.1.pre.
-
Invin almost 4 yearsGlad, I could help
-
Konstantin Kozirev almost 4 yearsThe use of MaterialApp is not justified in this case. It can be just
runApp(new myApp())
invoid main()
. If you use MaterialApp, then it will be in different uglish styling. -
Konstantin Kozirev almost 4 yearsMoreover, I just checked and it also works with
StatelessWidget
. So absolutely no reason to useStatefulWidget
andMaterialApp
. Hotreload works withStatelessWidget
. -
DYS over 3 yearsBig thanks! I doubt it's something to do with latest Flutter upgrade, only to find out your answer explains the culprit.
-
Bala over 3 yearsThis is the correct solution, if hot reload doesn't work even when hot reload preferences are enabled.
-
Petro about 3 yearsCan you explain more with some example code?
-
Kaigo almost 3 yearsDo you mean 'or' instead of 'of'?
-
Suj almost 2 yearsyes Invalidating caches fixes for me as well