Flutter) how to align bottom container inside listview
351
Solution 1
return Scaffold(
body: Stack(
children: [
Positioned(
bottom: 0,
width: MediaQuery.of(context).size.width,
child: Center(
child: MaterialButton(
child: Text("My button"),
onPressed: () {},
color: Colors.red,
),
),
),
ListView(
children: [
Text(
'check up',
style: TextStyle(
fontSize: 35,
fontWeight: FontWeight.bold,
color: Colors.black,
),
),
SizedBox(height: 12),
Text(
'SachsenwaldStr. 3. 12157 Berlin',
style: TextStyle(
fontSize: 20,
color: Colors.black,
),
),
],
)
],
));
Solution 2
You can use Scaffold Properties to make a button centre at the bottom. Hope it would be helpful for you,Thanks
import 'package:flutter/material.dart';
class BottomButton extends StatefulWidget {
const BottomButton({Key? key}) : super(key: key);
@override
_BottomButtonState createState() => _BottomButtonState();
}
class _BottomButtonState extends State<BottomButton> {
@override
Widget build(BuildContext context) {
return Scaffold(
///you can make the centre button use floating action Button and
provide its location
floatingActionButton: FloatingActionButton(
backgroundColor: Colors.transparent,
child: Container(
width: MediaQuery.of(context).size.width,
color: Colors.green,
child: Text(
"Button")),
onPressed: () {},
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
appBar: AppBar(
title: Text("MyAppBar"),
),
body: ListView(
physics: ClampingScrollPhysics(),
children: [
Column(
children: [
Text(
'check up',
style: TextStyle(
fontSize: 35,
fontWeight: FontWeight.bold,
color: Colors.black,
),
),
SizedBox(height: 12),
Text(
'SachsenwaldStr. 3. 12157 Berlin',
style: TextStyle(
fontSize: 20,
color: Colors.black,
),
),
],
),
],
),
///bottom sheet place button at bottom without using space
bottomSheet: Container(
width: MediaQuery.of(context).size.width,
color: Colors.green,
child: TextButton(onPressed: () {}, child: Text("Button"))),
);
}
}
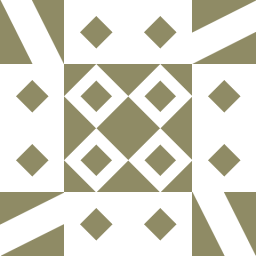
Author by
Noah_J.C_Lee
Updated on December 02, 2022Comments
-
Noah_J.C_Lee 28 days
Hi i am refactoring our code
and i has got some problems.
Scaffold( backgroundColor: Colors.white, appBar: buildAppBar(context, ''), body: ListView( physics: ClampingScrollPhysics(), children: [ Column( children: [ Text( 'check up', style: TextStyle( fontSize: 35, fontWeight: FontWeight.bold, color: Colors.black, ), ), SizedBox(height: 12), Text( 'SachsenwaldStr. 3. 12157 Berlin', style: TextStyle( fontSize: 20, color: Colors.black, ), ), ], ), Spacer(), buildNextButton(context), ], ),
so in need to put ,buildNextButton' bottom center but
Align( alignment: Alignment.bottomCenter, child: buildNextButton(context), ),
didn't work
and also Spacer() too
is there any nice ways too align buildNextButton at the bottom
-
Noah_J.C_Lee 12 monthsthx for you're anwser but it didn't work inside Listview...
-
Vaidarbhi 12 monthsPlease check my another answer which I post currently
-
Vaidarbhi 12 monthsWrap your listview with Stack and Position Widgets with fit and bottom properties respectively. :)
-
Noah_J.C_Lee 12 monthshm... then well I was keep using Stacks to position a button at the bottom. And was trying to replace Stacks to Listview and Do the samething... but seems theres no other ways to put a Container below only with ListView
-
Vaidarbhi 12 monthsNo no , don't replace stack to listview. Your code hierarchy will be body: Stack -> Position -> ElevatedButton -> Listview and so on.. Please allow me some minutes , I will back with your working code. :)