Flutter | How to store list of int in Shared preference
Solution 1
Currently, there is not any option to directly save the list of ints in Sharedpreferences. You can save the list of Strings in sharedpreferences.
The easiest way for your case is to convert your List<int> values to List<String> and store them.
Also, at the time of retrieval you can fetch the List<String> stored in shared prefs and convert it to your original List<int>
For Ex :
To store your list in shared prefs.
SharedPreferences prefs=await SharedPreferences.getInstance();
// your custom int list
List<int> mList=[0,1,2,3,4];
// convert your custom list to string list
List<String> stringsList= mList.map((i)=>i.toString()).toList();
// store your string list in shared prefs
prefs.setStringList("stringList", stringsList);
To retrieve & convert your stored string list to your original int list
SharedPreferences prefs=await SharedPreferences.getInstance();
// fetch your string list
List<String> mList = (prefs.getStringList('stringList') ?? List<String>());
//convert your string list to your original int list
List<int> mOriginaList = mList.map((i)=> int.parse(i)).toList();
print(mOriginaList);
Solution 2
You can copy paste run full code below
You can
Step 1: Convert List<int>
to List<String>
Step 2: Save with prefs.setStringList
Step 3: Get it back with prefs.getStringList
Step 4: Covert to List<int>
code snippet
List<int> intProductListOriginal = [11, 22, 33, 44, 55];
void _incrementCounter() async {
List<String> strList = intProductListOriginal.map((i) => i.toString()).toList();
SharedPreferences prefs = await SharedPreferences.getInstance();
prefs.setStringList("productList", strList);
List<String> savedStrList = prefs.getStringList('productList');
List<int> intProductList = savedStrList.map((i) => int.parse(i)).toList();
print("${intProductList.toString()}");
Output
I/flutter ( 4347): [11, 22, 33, 44, 55]
full code
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
List<int> intProductListOriginal = [11, 22, 33, 44, 55];
void _incrementCounter() async {
List<String> strList = intProductListOriginal.map((i) => i.toString()).toList();
SharedPreferences prefs = await SharedPreferences.getInstance();
prefs.setStringList("productList", strList);
List<String> savedStrList = prefs.getStringList('productList');
List<int> intProductList = savedStrList.map((i) => int.parse(i)).toList();
print("${intProductList.toString()}");
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headline4,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
),
);
}
}
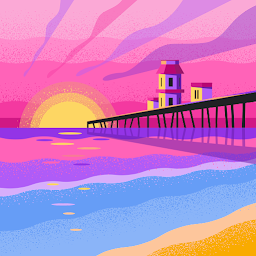
Comments
-
Rutvik Gumasana over 1 year
Here I want to store all the Ids of product in shared preference. but here when I am storing id using
setInt
it will store only one Id and remove previous IDs so I want that all id needs to be stored in shared preference and how to get all those ids that are stored in shared preferences.Here is the code I've tried
unAuthAddCart(dynamic itemId) async { try { SharedPreferences pref = await SharedPreferences.getInstance(); var unAuthItemId = pref.setInt('unAuthItemid', itemId); print(unAuthItemId); var abc = pref.getInt('unAuthItemid'); print("UnAuth Itme Id $abc"); } catch (e) { print(e); print("Something went Wrong in Particular Product Cart"); } }