Flutter show's a red error screen before loading data from firebase using StreamBuilder, how to fix?
Solution 1
So after trying out some stuff I finally fixed this error. decided to post an answer since this question doesn't have any solution online. at-least I couldn't find one. hope this helps people having the same issue
what I first did was declare a String which contained the value of user.uid
String userid;
void _getUser() async {
FirebaseUser user = await FirebaseAuth.instance.currentUser();
userid=user.uid;
}
now in the card builder widget I got rid of the extra stuff and simply called _getUser() method in the init state
class _CardBuilderState extends State<CardBuilder> {
@override
void initState() {
super.initState();
_getUser();
}
@override
Widget build(BuildContext context) {
return StreamBuilder(
stream: Firestore.instance.collection(UserInformation).document(userid).snapshots(),
builder: (context, snapshot) {
if(snapshot.hasData)
{
final userDocument = snapshot.data;
final title=userDocument[widget.text];
return Card(
margin: EdgeInsets.only(left: 20,right: 20,top: 20),
child: ListTile(
leading:
Icon(widget.icon, color: QamaiGreen, size: 20.0),
title: AutoSizeText(
'${widget.title} : $title',
style: TextStyle(
fontFamily: 'Montserrat',
fontWeight: FontWeight.w600,
color: QamaiThemeColor,
fontSize: 15
),
maxLines: 1,
overflow: TextOverflow.ellipsis,
maxFontSize: 15,
minFontSize: 9,
),
));
}
else{
return Card(
margin: EdgeInsets.only(left: 20,right: 20,top: 20),
child: ListTile(
leading:
Icon(widget.icon, color: QamaiGreen, size: 20.0),
title: AutoSizeText(
'LOADING...',
style: TextStyle(
fontFamily: 'Montserrat',
fontWeight: FontWeight.w600,
color: QamaiThemeColor,
fontSize: 15
),
maxLines: 1,
overflow: TextOverflow.ellipsis,
maxFontSize: 15,
minFontSize: 9,
),
));
}
});
}
}
Last changes were to add a condition checking if the snapshot has data or not. also saving the specific document field in a final variable helped a lot in my case that is
final title=userDocument[widget.text];
and finally an else condition was added to mimic the same card interface that shows a text which says loading. you can use CirculorIndicator if you want that will also work for me this was looking more natural for the interface.
Solution 2
you can show a progress indicator as your load your data as shown below
return StreamBuilder(
stream: Firestore.instance.collection(UserInformation).document(user.uid).snapshots(),
builder: (context, snapshot) {
//==========show progress============
if (!snapshot.hasData) {
return CircularProgressIndicator()
}
var userDocument = snapshot.data;
// User userDocument here ..........
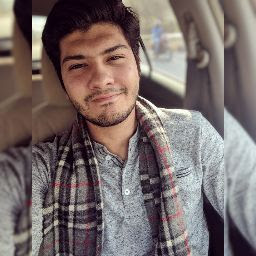
junaid tariq
Updated on December 14, 2022Comments
-
junaid tariq over 1 year
I am getting data from firebase and making cards using that data. to display certain data of user from database, the data loads fine but for 1 second or more there is a red error screen. I want this to go away I'll show you my code. can somebody please help show me what's wrong. I have no clue what I am doing wrong that this happens and I get the error
The getter 'uid' was called on null. Receiver: null Tried calling: uid
The method '[]' was called on null. Receiver: null Tried calling:
here is my code, any help will be appreciated thankyou
class CardBuilder extends StatefulWidget { final String title; final String text; final IconData icon; CardBuilder({Key key, this.title,this.text,this.icon}): super(key: key); @override _CardBuilderState createState() => _CardBuilderState(); } class _CardBuilderState extends State<CardBuilder> { FirebaseUser user; String error; void setUser(FirebaseUser user) { setState(() { this.user = user; this.error = null; }); } void setError(e) { setState(() { this.user = null; this.error = e.toString(); }); } @override void initState() { super.initState(); FirebaseAuth.instance.currentUser().then(setUser).catchError(setError); } @override Widget build(BuildContext context) { return StreamBuilder( stream: Firestore.instance.collection(UserInformation).document(user.uid).snapshots(), builder: (context, snapshot) { var userDocument = snapshot.data; // User userDocument here .......... return Card( margin: EdgeInsets.only(left: 20,right: 20,top: 20), child: ListTile( leading: Icon(widget.icon, color: QamaiGreen, size: 20.0), title: AutoSizeText( '${widget.title} : ${userDocument[widget.text]}', style: TextStyle( fontFamily: 'Montserrat', fontWeight: FontWeight.w600, color: QamaiThemeColor, fontSize: 15 ), maxLines: 1, maxFontSize: 15, minFontSize: 12, ), )); }); } }
-
junaid tariq over 4 yearsThanks for the reply, This is not working at all. has zero affect on the red screen
-
griffins over 4 yearsFork your question you're able to load the data but before the data is loaded you get a red screen , you can use a property of stream builder initial data
-
griffins over 4 yearsTo set initial data
-
griffins over 4 yearsAlthough what I have in my answer has been working great for me
-
junaid tariq over 4 yearsI'm not sure what to set in the initial data, would you have any suggestions?