Flutter sqflite insert List<String>
I'm pretty sure you cannot store a list of strings in this way. According to the docs, it only supports a blob of ints. I think there are two ways you could do this.
One way would be to stringify the answers into a single string. You can use the join method and separate it by a character that no answer will contain (maybe a pipe like |). Then, when you read from the database, you can split the string back into an array using the character you chose.
The other way to do this would be to create a table that associates a single question with multiple answers. This is basically sudo code, but if you had a table that matched this it should work.
Answer
int id autoincremented, int questionId, String answerText, int isCorrectAnswer (0 or 1)
Also, a bit unrelated, but sqflite supports the AUTOINCREMENT keyword which I recommend you use for your primary key IDs.
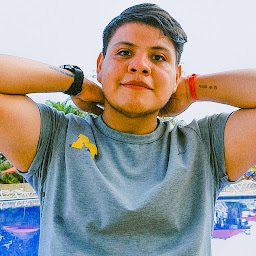
Bryansq2nt
Updated on December 15, 2022Comments
-
Bryansq2nt over 1 year
i'm trying to insert a List into a sql database in flutter , but i dont know how can i do it , can anyone help me ?
i have this when i initialize mi database:
Directory documentsDirectory = await getApplicationDocumentsDirectory(); String path = join(documentsDirectory.path, "tuCuestionarioDB.db"); return await openDatabase(path, version: 1, onOpen: (db) {}, onCreate: (Database db, int version) async { await db.execute("CREATE TABLE Question (" "id INTEGER PRIMARY KEY," "subject INTEGER," "topic INTEGER," "question TEXT," "answer INTEGER," "answers TEXT ARRAY," //THIS IS MY LIST<STRING> "rightAnswer INTEGER," "correctly BIT," "answered BIT" ")"); });
and i have this to insert data:
newQuestion(Question newQuestion) async { final db = await database; //get the biggest id in the table var table = await db.rawQuery("SELECT MAX(id)+1 as id FROM Question"); int id = table.first["id"]; //insert to the table using the new id var raw = await db.rawInsert( "INSERT Into Question (id,subject,topic,question,answer,answers,rightAnswer,correctly,answered)" " VALUES (?,?,?,?,?,?,?,?,?)", [id != null ? id : 0, newQuestion.subject, newQuestion.topic,newQuestion.question,newQuestion.answer,newQuestion.answers,newQuestion.rightAnswer,newQuestion.correctly ? 1 : 0,newQuestion.answered ? 1 : 0]); return raw; }
but when y try to insert a value like this:
{subject: 1, topic: 1, question: What is the best community?, answer: 3, rightAnswer: 0, answers: [Stack Overflow, Facebook, Yahoo Answers], correctly: false, answered: false}
i got a error like this:
Exception has occurred.
SqfliteDatabaseException (DatabaseException(java.lang.String cannot be cast to java.lang.Integer) sql 'INSERT Into Question (id,subject,topic,question,answer,answers,rightAnswer,correctly,answered) VALUES (?,?,?,?,?,?,?,?,?)' args [0, 1, 1, What is the best community?, 3, [Stack Overflow, Facebook, Answers Yahoo], 0, 0, 0]})
and when i remove anwsers field i got no erros