Flutter throws an error when i use Text field with auto focus
Solution 1
This issue exists even in official example https://flutter.dev/docs/cookbook/forms/focus
Because with auto focus, the keyboard show up first but textfield is still not exist
Workaround is use FocusNode
and WidgetsBinding.instance.addPostFrameCallback
When TextField show and then move focus to this TextField
code snippet
@override
void initState(){
super.initState();
myFocusNode = FocusNode();
WidgetsBinding.instance.addPostFrameCallback((_){
FocusScope.of(context).requestFocus(myFocusNode);
});
}
full code
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
FocusNode myFocusNode;
@override
void initState(){
super.initState();
myFocusNode = FocusNode();
WidgetsBinding.instance.addPostFrameCallback((_){
FocusScope.of(context).requestFocus(myFocusNode);
});
}
@override
void dispose() {
// Clean up the focus node when the Form is disposed.
myFocusNode.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: TextField(
//autofocus: true,
focusNode: myFocusNode,
),
),
);
}
}
Solution 2
This bug has been fixed, but as yet, the fix is not in the stable channel (https://github.com/flutter/flutter/issues/52221). If your project allows, you can switch to the dev channel (>1.15) and just use the autofocus.
From the command line:
flutter channel dev
flutter upgrade
Rebuild your project, and it should work.
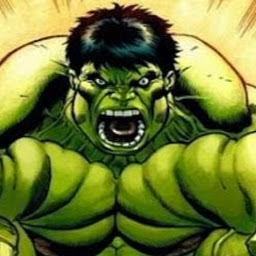
josef atef
Updated on December 16, 2022Comments
-
josef atef over 1 year
When i use a TextField widget in Flutter and set the value of the propriety autoFocus to true
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State<MyApp> { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: TextField( autofocus: true, ), ), ); } }
flutter throws an error
I/flutter (31721): ══╡ EXCEPTION CAUGHT BY FOUNDATION LIBRARY ╞════════════════════════════════════════════════════════ I/flutter (31721): The following assertion was thrown while dispatching notifications for FocusNode: I/flutter (31721): RenderBox was not laid out: RenderEditable#bf516 NEEDS-LAYOUT NEEDS-PAINT I/flutter (31721): 'package:flutter/src/rendering/box.dart': I/flutter (31721): Failed assertion: line 1687 pos 12: 'hasSize' I/flutter (31721): I/flutter (31721): Either the assertion indicates an error in the framework itself, or we should provide substantially I/flutter (31721): more information in this error message to help you determine and fix the underlying cause. I/flutter (31721): In either case, please report this assertion by filing a bug on GitHub: I/flutter (31721): https://github.com/flutter/flutter/issues/new?template=BUG.md I/flutter (31721): I/flutter (31721): When the exception was thrown, this was the stack: I/flutter (31721): #2 RenderBox.size package:flutter/…/rendering/box.dart:1687 I/flutter (31721): #3 EditableTextState._updateSizeAndTransform package:flutter/…/widgets/editable_text.dart:1729 I/flutter (31721): #4 EditableTextState._openInputConnection package:flutter/…/widgets/editable_text.dart:1415 I/flutter (31721): #5 EditableTextState._openOrCloseInputConnectionIfNeeded package:flutter/…/widgets/editable_text.dart:1441 I/flutter (31721): #6 EditableTextState._handleFocusChanged package:flutter/…/widgets/editable_text.dart:1707 I/flutter (31721): #7 ChangeNotifier.notifyListeners package:flutter/…/foundation/change_notifier.dart:206 I/flutter (31721): #8 FocusNode._notify package:flutter/…/widgets/focus_manager.dart:808 I/flutter (31721): #9 FocusManager._applyFocusChange package:flutter/…/widgets/focus_manager.dart:1401 I/flutter (31721): (elided 12 frames from class _AssertionError and package dart:async) I/flutter (31721): I/flutter (31721): The FocusNode sending notification was: I/flutter (31721): FocusNode#ce6fe I/flutter (31721): ════════════════════════════════════════════════════════════════════════════════════════════════════
Why , and how can i solve this problem.
my flutter version :
[√] Flutter (Channel stable, v1.12.13+hotfix.5, on Microsoft Windows [Version 10.0.18362.535], locale en-US) [√] Android toolchain - develop for Android devices (Android SDK version 29.0.2) [√] Android Studio (version 3.5) [√] VS Code (version 1.41.0) [√] Connected device (1 available) • No issues found!
========================================================================