Flutter type '_InternalLinkedHashMap<String, dynamic>' is not a subtype of type 'List<dynamic>'
You have incorrectly cast the "USDBRL" as a List, when it is a Map.
Get rid of this line:
List<dynamic> body = json["USDBRL"];
and replace with this line:
Map<String, dynamic> body = json["USDBRL"];
That should resolve the casting error you are seeing.
To resolve the toList
error, you need to change how you are getting the Dolar
.
Dolar dolar = Dolar.fromJson(body);
This is because the "USDBRL" does not contain a list of items. It is one object with properties and values. I'm assuming that those values inside "USDBRL" are what you are wanting to use to configure the data in the Dolar object. So you just change it to be a single instance of Dolar
that gets it's data from the "USDBRL" Map.
The final code could look something like this:
Future<Dolar> getDolar() async {
try {
Response res = await get(Uri.parse(endPointUrl));
if (res.statusCode==200) {
final json = jsonDecode(res.body);
Dolar dolar = Dolar.fromJson(json["USDBRL"] as Map<String, dynamic>);
return dolar;
} else {
throw ("Can't access the Dolar API");
}
} catch (e) {
print(e);
rethrow;
}
}
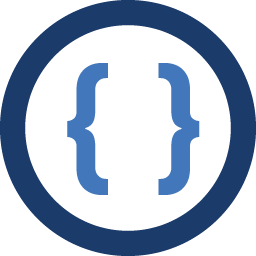
Admin
Updated on January 04, 2023Comments
-
Admin over 1 year
I'm a Flutter language learner, new to this world,
{ "USDBRL": { "id": "...", "name": "...",... } }
My Dolar Model:
class Dolar { String param1; String param2; // Constructor Dolar({ required this.param1, required this.param2, }); factory Dolar.fromJson(Map<String, dynamic> json) { return Dolar( param1: json['param1'], param2: json['param2'], ); } }
I need to grab all "USDBRL" fields, but when I run the app I get "flutter: type '_InternalLinkedHashMap<String, dynamic>' is not a subtype of type 'List<dynamic>' ".
I've tried searching for resolutions on the internet, but none of the alternatives I've tried have worked.
-
Admin about 2 yearsError: The method 'toList' isn't defined for the class 'Map<dynamic, dynamic>'. lib/services/api_service.dart:21 - 'Map' is from 'dart:core'. Try correcting the name to the name of an existing method, or defining a method named 'toList'. body.map((dynamic item) => Dolar.fromJson(item)).toList(); ^^^^^^
-
daddygames about 2 yearsYou may need to update your question to include the Dolar model and see the
toList
function that you are attempting to access. -
Admin about 2 yearsI put an example equal to my Model and a print of the current error after your suggestion
-
daddygames about 2 yearsAre you attempting to get the
Dolar
data out of the "USDBRL" object? -
Admin about 2 yearsYes, all of them, id, name, as it is in the json template I put above, to put them in my model, which has the same fields
-
daddygames about 2 yearsUpdated the answer to address the issue
-
Admin about 2 yearsI didn't understand, sorry
-
daddygames about 2 yearsI updated the answer again to try to clarify. It includes a representation of what your code could potentially look like combining the concepts I have tried to explain.
-
Admin about 2 yearsThank you very much, my problem was solved at first.
-
Admin about 2 yearsThe answer from daddy Games above already solved my problem, I also appreciate your help :D