Flutter unable to catch PlatformException while attempting firebase auth
Solution 1
I was having the same issue for a while and found out the reason why.
The issue here is, although it "seems" you cannot catch the PlatformException , in reality, you "are catching" the exception.
In Android Studio, we can set the debug breaking policy,
by going to Run > View Breakpoints.
If you uncheck the Enabled, you will find out that the Android Studio "doesn't get in the way" of your catching the exception and your app will display the snackbar properly when catch clause executes which is what we expect "when we are not debugging" the app.
But when we are debugging with the Break on exceptions enabled, Android Studio simply does its job by breaking on PlatformException before the catch clause executes.
So, you could just uncheck the Enabled option or just press the "Resume Program" button in Android Studio and just not worry about it. You're app is probably working just fine. :)
Solution 2
I have also recently faced this error and, I have found out that the .catchError()
callback wasn't being called in the debug mode (which is when you click the Run->Start Debugging
button in VSCode).
However, when you type in flutter run -d , then, the .catchError()
method gets called back as it is not in debug mode.
To get your preferred simulator's code paste this line of code in the terminal:
instruments -s devices
If that doesn't work, you can also try pasting this:
xcrun simctl list
The .catchError()
method will get called unlike before and the code inside that will get executed as expected!
Additionally, the app won't crash anymore with a PlatformException()
and instead you will get a log like this one:
[VERBOSE-2:ui_dart_state.cc(157)] Unhandled Exception: NoSuchMethodError: The getter 'uid' was called on null.
Receiver: null
I have been facing this problem in Google Sign In too, in which the .catchError()
was not being called!
In conclusion, if you have some error with handling errors in Firebase Authentication, you should first try to first run in through the terminal. Thanks, and I hope this helps!
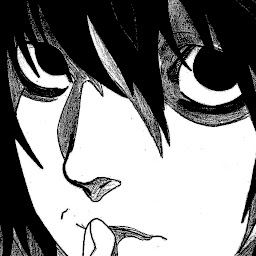
Robin Olisa
Updated on December 05, 2022Comments
-
Robin Olisa over 1 year
I just recently began using flutter, I am trying to implement firebase signing in using email and password and it works when I input the correct email and password but not when the password is wrong. It gives a PlatformException when the password is wrong and then the app freezes even though I've tried to catch the exception while android studio shows this below:
Here is my sign in implementation:
String _reason; Future<void> _performLogin() async { String reason; // This is just a demo, so no actual login here. try{ FirebaseUser user = await FirebaseAuth.instance.signInWithEmailAndPassword(email: _email, password: _password); if(user != null){ Navigator.of(context).push(new MaterialPageRoute<dynamic>( builder: (BuildContext context) { return new MyApp(); }, )); final snack = new SnackBar( content: new Text("Signed In Successfully"), action: null, duration: new Duration(seconds: 4), backgroundColor: Colors.black, ); Scaffold.of(context).showSnackBar(snack); } }on PlatformException catch(e){ reason = '${e.message}'; /*final snack = new SnackBar( content: new Text(e.message), action: null, duration: new Duration(seconds: 4), backgroundColor: Colors.black, ); Scaffold.of(context).showSnackBar(snack);*/ } setState(() { _reason = reason; }); }
I also added
import 'package:flutter/services.dart' show PlatformException;
Please let me know how to catch the exception, thank you.