flutter_map zoom not updating
You can copy paste run full code below
You can use MapController
and call mapController.move(currentCenter, currentZoom)
code snippet
double currentZoom = 13.0;
MapController mapController = MapController();
LatLng currentCenter = LatLng(51.5, -0.09);
void _zoom() {
currentZoom = currentZoom - 1;
mapController.move(currentCenter, currentZoom);
}
working demo
full code
import 'package:flutter/material.dart';
import 'package:flutter_map/flutter_map.dart';
import 'package:latlong/latlong.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
double currentZoom = 13.0;
MapController mapController = MapController();
LatLng currentCenter = LatLng(51.5, -0.09);
void _zoom() {
currentZoom = currentZoom - 1;
mapController.move(currentCenter, currentZoom);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: FlutterMap(
mapController: mapController,
options: MapOptions(
center: currentCenter,
onTap: (pos) {
print(pos);
},
zoom: currentZoom,
// debug: true,
),
layers: [
TileLayerOptions(
overrideTilesWhenUrlChanges: false,
urlTemplate:
"https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png?source=${DateTime.now().millisecondsSinceEpoch}",
subdomains: ['a', 'b', 'c'],
additionalOptions: {},
)
]),
floatingActionButton: FloatingActionButton(
onPressed: _zoom,
tooltip: 'Zoom',
child: Icon(Icons.remove_circle_outline_rounded),
),
);
}
}
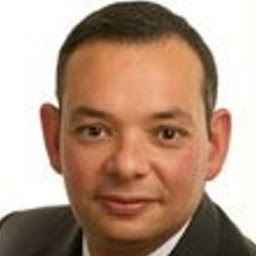
Emmanuel Cohen-Laroque
Updated on December 24, 2022Comments
-
Emmanuel Cohen-Laroque over 1 year
I am using the flutter_map plugin with OpenStreetMap as a provider. I have two buttons for changing zoom level, that increase/decrease the currentZoom variable (double).
In the build override of my widget, I am rebuilding the map as follow:
@override Widget build(BuildContext context) { FlutterMap currentmap = FlutterMap( options: MapOptions( center: LatLng(latitude, longitude), onTap: (pos) { print(pos); }, zoom: currentZoom, // debug: true, ), layers: [ TileLayerOptions( overrideTilesWhenUrlChanges: false, urlTemplate: "https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png?source=${DateTime.now().millisecondsSinceEpoch}", subdomains: ['a', 'b', 'c'], additionalOptions: {}, ),
, then currentMap is used as the child of a canvas, which does work perfectly.
Please notice I have added a ?source=at the end of the URL to force cache update (but removing this argument does not solve the problem neither).
However, when I change the currentZoom level in the buttons onPressed callbacks and then call SetState, the page is rebuilt but the map zoom level does not change at all.
Here is the callback:
IconButton(icon: Icon(Icons.remove_circle_outline_rounded), onPressed: () => setState(() { currentZoom = currentZoom < 1 ? 0 : currentZoom - 1; print(currentZoom); }), ),
In the callbacks, I have console logs that are showing the currentZoom variable is correctly updated.
Am I missing something ?
-
Emmanuel Cohen-Laroque over 3 yearsThanks a lot! I was indeed missing the MapController api. Cheers.