For Loop to Count Letters in a Word
Solution 1
It seems like you don't understand the difference between print and return. What you are doing is printing every single occurrence in the loop, when what you want is the final value at the end. What you would have to do is keep the same code, except when the loop terminates, you want to return whatever value is at the end. So get rid of print count, and remove the indent. Then you must return count. You don't want to return in the loop, because this will return the first value of count after a single character is checked. Message me for further questions if you have any.
Solution 2
Just change:
for char in a:
if char == 'a':
count+=1
print count
To:
for char in a:
if char == 'a':
count+=1
print count
Otherwise you'll be printing the counter each time the for loop runs.
Also, you're calling your function with print at print count_letters(a)
.
You don't need this since you've put a print statement at the last line in your code. If you left the print
statement there to call your function, it'll print None
, since the function already have returned the count value.
So you can also change:
print count_letters(a)
To:
count_letters(a)
Output Example with print count_letters(a)
:
Name a fruit and I will tell you how many letters are in it. abacaxi
3
None
Output Example with count_letters(a)
:
Name a fruit and I will tell you how many letters are in it. abacaxi
3
Solution 3
If you want to keep a similar structure and calculate the count yourself, you can use :
def count_a(fruit_name):
return sum(1 for char in fruit_name if char == 'a')
It's shorter, it's more pythonic, it's not a standard method call, it returns an integer instead of just printing it and the variable names might be a bit clearer.
If you don't mind using an existing python method, you can use :
return fruit_name.count('a')
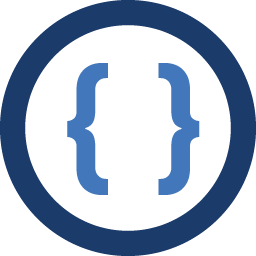
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm just learning python and I came to a problem that required me to put a string as an input in my "count letters function."
The function is supposed to count how many
"a"s
are in a word given (specifically, a fruit inputed by the user).The final value is correct, but in my function, it lists me the programs "procedure" if you will by listing how many
"a"s
are at each index and adding everything that was before.I just want one final value, not a list.
If I'm not too clear, here is my program and test it out for yourself to see what I mean, and maybe help me come to a solution?
def count_letters(a): count=0 for char in a: if char == 'a': count+=1 print count a=raw_input("Name a fruit and I will tell you how many letters are in it." + '\n') print count_letters(a)