Force iOS app to launch in landscape mode
Solution 1
In your application’s Info.plist file, add the UIInterfaceOrientation
key and set its value to the
landscape mode. For landscape
orientations, you can set the value
of this key to
UIInterfaceOrientationLandscapeLeft
or
UIInterfaceOrientationLandscapeRight
.
Lay out your views in landscape mode and make sure that their autoresizing options are set correctly.
Override your view controller’s shouldAutorotateToInterfaceOrientation:
method and return YES only for the
desired landscape orientation and NO
for portrait orientations.
Solution 2
use the following in appDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
[[UIApplication sharedApplication] setStatusBarOrientation:UIInterfaceOrientationLandscapeRight];
also set the required orientations in
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation
{
// Return YES for supported orientations
return (interfaceOrientation == UIInterfaceOrientationLandscapeRight);
}
//Here are some iOS6 apis, used to handle orientations.
- (BOOL)shouldAutorotate NS_AVAILABLE_IOS(6_0)
- (NSUInteger)supportedInterfaceOrientations - (UIInterfaceOrientation)preferredInterfaceOrientationForPresentation
Solution 3
When you click on your project's name in XCode and select your target under TARGETS, under Summary tab there's a visual representation of the supported device orientation settings. That was how I managed to do it.
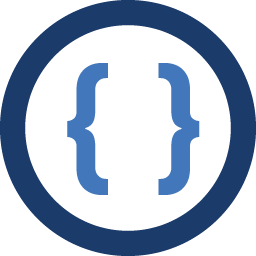
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am developing an app for iOS 5, which have to run in landscape mode. My problem is that I cannot get it to flip initially.
I have tried the adding "Initial interface orientation" set to "Landscape (right home button)" and adding the following method to my view controller:
- (BOOL)shouldAutorotateToInterfaceOrientation:(UIInterfaceOrientation)interfaceOrientation { // Return YES for supported orientations return (interfaceOrientation == UIInterfaceOrientationLandscapeRight); }
I can wrap my head around how it is supposed to work. (I found the code here)
I am also wondering how to use the "Supported Device Orientation" available in the Xcode 4.2 project setup, it does not seem to do anything.
I have been looking around the website and have not been able to find an example that solves my problem.
Thank you.
-
Admin over 12 yearsThank you. I did do as you described. I have more then one subview and found out I had to override the method on all controllers before I got it to work.
-
Jignesh B over 10 yearsshouldAutorotateToInterfaceOrientation: not called help me its appreciated
-
vishy over 10 years@JigPatel use some of these new methods in ios6
- (BOOL)shouldAutorotate NS_AVAILABLE_IOS(6_0) - (NSUInteger)supportedInterfaceOrientations - (UIInterfaceOrientation)preferredInterfaceOrientationForPresentation
-
Avi Cohen over 9 yearsif in your info.plist you put the UIInterfaceOrientationLandscapeRight above the other landscapes then you can start your app on landscape