Foreach giving undefined variable error
Solution 1
You need to check to see if the entry name is set in your multidimensional array. Here is the long version because I was unaware of the short_open_tags
option in PHP.
Code
<?
$entrees = array();
$entrees[0]['name']="Lamb";
$entrees[0]['description'] ="A small leg of lamb";
$entrees[1]['name']="Ham";
$entrees[1]['description'] ="Large thing of ham";
echo "<ul>\n";
foreach ($entrees as $entree) {
if(!empty($entree['name'])){
echo "<li>";
echo "<span class=\"food-name\">";
echo $entree['name'];
echo "</span>";
echo "</li>\n";
}
}
echo "</ul>";
?>
Output
<ul>
<li><span class="food-name">Lamb</span></li>
<li><span class="food-name">Ham</span></li>
</ul>
Update
Learned something new thanks to @Arjan - the original code you had with the [short_open_tags][1]
should also work with the if
statement provided they are enabled in your build. In my testing they threw an error as my test machine uses an older build of PHP. After rebuilding they do work by default.
<?=$entree['name']?>
According to the manual in this usage the =
sign means echo()
and is enabled by default in PHP 5.4.1. Upon searching through Google because their searches use the operators differently I was unable to find a link since I did not know the name for the usage. If you're programming with a team of developers make sure to use the same agreed upon tag styles as the rest of the team.
Solution 2
You need to set short_open_tag
to On
in php.ini. That fixed it for me with no additional code.
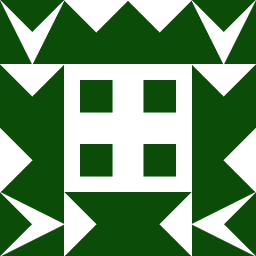
Dylan Buth
Updated on June 04, 2022Comments
-
Dylan Buth 7 months
Bare in mind that it's late where I am so this may just be a very simple solution that I am overlooking.
I have this foreach statement:
<? foreach ($entrees as $entree) { ?> <li> <span class="food-name"> <?=$entree['name']?> </span> </li> <?}?>
That gives an Undefined variable error for $entree.
A PHP Error was encountered Severity: Notice Message: Undefined variable: entree Filename: views/welcome_message.php Line Number: 9
I tried
print_r($entrees);
and the result wasArray ( [0] => Array ( [name] => Lamb [description] => A small leg of lamb ) [1] => Array ( [name] => Ham [description] => Large thing of ham ) ) 1
Again, this may be something stupid simple but I've been trying to 30 min and can't get it to work for the life of me. Thanks in advance for the help.
-
Dylan Buth over 9 yearsIt sort of works. It repeats the first result. What is this tArr? Can't find it on google.
-
AbsoluteƵERØ over 9 yearsIt's just a placeholder short for temporary array. We're assigning the key to
$entree
and the value array associated with the$key
to$tArr
. -
AbsoluteƵERØ over 9 yearsEh, I've updated my code. It should be easier for you to follow since it's the style you're used to.
-
Dylan Buth over 9 yearsI was just renaming variables as a test. Copied the wrong error message.
-
AbsoluteƵERØ over 9 yearsThat works. Some of my accounts are on Dreamhost and they have some strange errors they've compiled in from time to time. "Error: You are not a Jedi." Their server names are strange too... things like
eggnog
. -
Dylan Buth over 9 yearsWhen I tried
<? echo $entree['name']; ?>
it showed in DOM as a comment. When I did same thing with<?php ?>
I got the same undefined variable error. -
AbsoluteƵERØ over 9 yearsI've added an if statement to make sure there is a variable there. If there isn't in your multidimensional array it will throw an undefined index error. The DOM is the Document Object Model. It might have said that if there aren't any <ul> or <ol> tags.
-
Dylan Buth over 9 yearsI am confused by your code though. It's great that is works but I don't understand it. Like the \n and class=\"food-name\">"
-
AbsoluteƵERØ over 9 yearsSince I've putting everything into quotes I have to escape the same quotes. The
\n
is a new line character (to make it easier to read in HTML). -
Dylan Buth over 9 yearsSo could the same be accomplished with
class='food-name'
? -
AbsoluteƵERØ over 9 yearsYes. As long as the quotes do not match the container they do not need to be escaped.
-
Arjan over 9 yearsActually,
<?=$foo;?>
is the same as<?php echo $foo; ?>
ifshort_open_tags
are enabled. -
AbsoluteƵERØ over 9 years@Arjan Thanks for that. I tried searching for the equal signs but Google returns no results (probably by design). google.com/…
-
user2580401 over 4 yearsOMG this exact problem has caught me so off guard. Thanks for the tip! (Even if it's been 4 years since you posted it). To other googlers: an alternative to this solution is to replace actual short open tags (
<?
) with normal ones (<?php
) in your code.