format ssn using regex
Solution 1
You're using last
as a named group in your replacement string without specifying it in your pattern.
Update your pattern as follows and your existing code should work:
(?:[0-9]{3})(?:[0-9]{2})(?<last>[0-9]{4})
Depending on your input you may want to restrict the pattern to match the entire input by using the ^
and $
metacharacters to match the start and end of the string, respectively. By doing so the regex won't match an input with more than 9 consecutive numbers. This would look like:
^(?:[0-9]{3})(?:[0-9]{2})(?<last>[0-9]{4})$
Also, since all you care about is the last 4 digits, you might choose to match 5 numbers, followed by 4 numbers, instead of splitting it up into 3 groups:
^(?:[0-9]{5})(?<last>[0-9]{4})$
In addition, if your input is always 9 numbers and can be trusted, then regex is not needed. You could simply get the substring to extract the last 4 characters:
string SSN = "123456789";
string formattedSSN = "XXX-XX-" + SSN.Substring(SSN.Length - 4, 4);
Solution 2
Generic code for SSN Masking.
string originalSSN = Convert.ToString("123-456-789").PadLeft(9, '0');
int maskDigit = 6;
string maskSSN = originalSSN.Substring(originalSSN.Length - maskDigit, maskDigit);
if (Regex.IsMatch(maskSSN, "(—)|(–)|(-)"))
{
int i = maskDigit;
while (Regex.Replace(maskSSN, "(—)|(–)|(-)", "").Length < maskDigit)
{
i++;
maskSSN = originalSSN.Substring(originalSSN.Length - i, i);
}
string[] ssnArray = Regex.Split(maskSSN, "(—)|(–)|(-)", RegexOptions.ExplicitCapture);
if (ssnArray.Length > 1)
{
maskSSN = originalSSN.Substring(originalSSN.Length - maskDigit - (ssnArray.Length - 1), maskDigit + (ssnArray.Length - 1));
}
}
maskSSN = maskSSN.PadLeft(9, '#');
Solution 3
How about this nice little function that will mask Tins as well as Ssns:
public static string MaskSSN(string originalSSN)
{
if (originalSSN.Length < 5) return originalSSN;
var trailingNumbers = originalSSN.Substring(originalSSN.Length - 4);
var leadingNumbers = originalSSN.Substring(0, originalSSN.Length - 4);
var maskedLeadingNumbers = Regex.Replace(leadingNumbers, @"[0-9]", "X");
return maskedLeadingNumbers + trailingNumbers;
}
So "123-45-6789" becomes "XXX-XX-6789" and "12-1234567" becomes "XX-XXX4567".
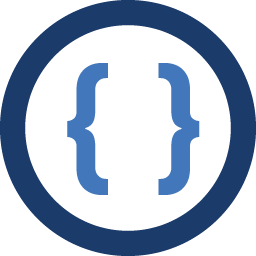
Admin
Updated on July 12, 2022Comments
-
Admin almost 2 years
Hi I need regex in c sharp to display SSN in the format of xxx-xx-6789. i.e 123456789 should be displayed as xxx-xx-6789 in a textfield. The code I am using write now is
string SSN = "123456789"; Regex ssnRegex = new Regex("(?:[0-9]{3})(?:[0-9]{2})(?:[0-9]{4})"); string formattedSSN = ssnRegex.Replace(SSN, "XXX-XX-${last}");
What is correct Reg Expression to mask ssn xxx-xx-6789 ?