Format the color of a cell in a pandas dataframe according to multiple conditions
25,413
Use applymap
:
from datetime import datetime, timedelta
import pandas as pd
name = ['Diego', 'Luis', 'Vidal', 'John', 'Yusef']
id = ['b000000005', 'b000000015', 'b000000002', 'b000000011', 'b000000013']
cel = [7878, 6464, 1100, 4545, 1717]
date = pd.to_datetime(['2017-05-31 20:53:00', '2017-05-11 20:53:00', '2017-05-08 20:53:00',
'2017-06-06 20:53:00', '2017-06-06 20:53:00'])
df = pd.DataFrame({'Name':name,'ID':id,'Cel':cel,'Date':date})
def color(val):
if val < datetime.now():
color = 'green'
elif val > datetime.now():
color = 'yellow'
elif val > (datetime.now() + timedelta(days=60)):
color = 'red'
return 'background-color: %s' % color
df.style.applymap(color, subset=['Date'])
Screenshot from Jupyter notebook. If you print
the output instead, you'll just get a reference to the Styler
object:
print(df.style.applymap(color, subset=['Date']))
<pandas.formats.style.Styler object at 0x116db43d0>
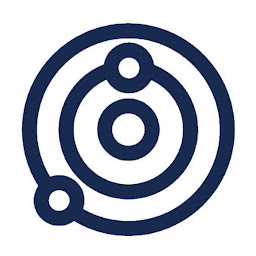
Author by
Yusef Jacobs
Updated on May 24, 2020Comments
-
Yusef Jacobs almost 4 years
I am trying to format the color of a cell of an specific column in a data frame, but I can't manage to do it according to multiple conditions.
This is my dataframe (df):
Name ID Cel Date 0 Diego b000000005 7878 2565-05-31 20:53:00 1 Luis b000000015 6464 2017-05-11 20:53:00 2 Vidal b000000002 1100 2017-05-08 20:53:00 3 John b000000011 4545 2017-06-06 20:53:00 4 Yusef b000000013 1717 2017-06-06 20:53:00
I want the values in the "Date" column to change color according to the following conditions:
if date < datetime.now(): color = 'green' elif date > datetime.now(): date = 'yellow' elif date > (datetime.now() + timedelta(days=60)): color = 'red'
This is my current code:
def color(val): if val < datetime.now(): color = 'green' elif val > datetime.now(): color = 'yellow' elif val > (datetime.now() + timedelta(days=60)): color = 'red' return 'background-color: %s' % color df.style.apply(color, subset = ['Fecha'])
I am getting the following error:
ValueError: ('The truth value of a Series is ambiguous. Use a.empty, a.bool(), a.item(), a.any() or a.all().', 'occurred at index Fecha')
The output is:
Out[65]: <pandas.formats.style.Styler at 0x1e3ab8dec50>
Any help will be appreciated.