Fortran Error Meanings
I think I can spot a few serious errors in your code sample. The syntax error is that you have unbalanced parentheses in the exp(...
statements. They should be like this:
tempi = exp(linear_interpolation(alt, temp, size(alt), alti) ) ! <- extra ")"
nuei = exp(linear_interpolation(alt, nue, size(alt), alti) )
oxyi = exp(linear_interpolation(alt, oxy, size(alt), alti) )
It's precisely things like this that can produce long strings of confusing errors like you're getting; therefore the advice Dave and Jonathan have given can't be repeated often enough.
Another error (the "unclassifiable statement") applies to your loops:
do(i=1, nend)
! ...
do(z=1, 100, dz)
! ...
These should be written without parentheses.
The "data declaration error" stems from your attempt to declare and initialise multiple variables like
real dz = size(alt)/100, z, integral = 0
Along with being positioned incorrectly in the code (as noted), this can only be done with the double colon separator:
real :: dz = size(alt)/100, z, integral = 0
I personally recommend always writing declarations like this. It must be noted, though, that initialising variables like this has the side effect of implicitly giving them the save
attribute. This has no effect in a main program, but is important to know; you can avoid it by putting the initialisations on a separate line.
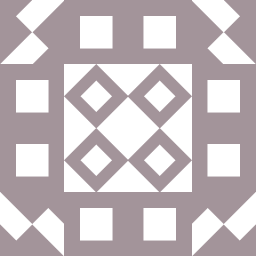
VashElric
Updated on June 04, 2022Comments
-
VashElric about 1 year
I have been following books and PDFs on writing in FORTRAN to write an integration program. I compile the code with gfortran and get several copies of the following errors.
1)Unexpected data declaration statement at (1) 2)Unterminated character constant beginning at (1) 3)Unclassifiable statement at (1) 4)Unexpected STATEMENT FUNCTION statement at (1) 5)Expecting END PROGRAM statement at (1) 6)Syntax error in data declaration at (1) 7)Statement function at (1) is recursive 8)Unexpected IMPLICIT NONE statement at (1)
I do not know hat they truly mean or how to fix them, google search has proven null and the other topics on this site we about other errors. for Error 5) i put in Program main and end program main like i might in C++ but still got the same result. Error 7) makes no sense, i am trying for recursion in the program. Error 8) i read implicit none was to prevent unnecessary decelerations.
Ill post the code itself but i am more interested in the compiling errors because i still need to fine tune the array data handling, but i cant do that until i get it working.
Program main implicit none real, dimension(:,:), allocatable :: m, oldm real a integer io, nn character(30) :: filename real, dimension(:,:), allocatable :: alt, temp, nue, oxy integer locationa, locationt, locationn, locationo, i integer nend real dz, z, integral real alti, tempi, nuei, oxyi integer y, j allocate( m(0, 0) ) ! size zero to start with? nn = 0 j = 0 write(*,*) 'Enter input file name: ' read(*,*) filename open( 1, file = filename ) do !reading in data file read(1, *, iostat = io) a if (io < 0 ) exit nn = nn + 1 allocate( oldm( size(m), size(m) ) ) oldm = m deallocate( m ) allocate( m(nn, nn) ) m = oldm m(nn, nn) = a ! The nnth value of m deallocate( oldm ) enddo ! Decompose matrix array m into column arrays [1,n] write(*,*) 'Enter Column Number for Altitude' read(*,*) locationa write(*,*) 'Enter Column Number for Temperature' read(*,*) locationt write(*,*) 'Enter Column Number for Nuetral Density' read(*,*) locationn write(*,*) 'Enter Column Number for Oxygen density' read(*,*) locationo nend = size(m, locationa) !length of column #locationa do i = 1, nend alt(i, 1) = m(i, locationa) temp(i, 1) = log(m(i, locationt)) nue(i, 1) = log(m(i, locationn)) oxy(i, 1) = log(m(i, locationo)) enddo ! Interpolate Column arrays, Constant X value will be array ALT with the 3 other arrays !real dz = size(alt)/100, z, integral = 0 !real alti, tempi, nuei, oxyi !integer y, j = 0 dz = size(alt)/100 do z = 1, 100, dz y = z !with chopped rounding alt(y) will always be lowest integer for smooth transition. alti = alt(y, 1) + j*dz ! the addition of j*dz's allow for all values not in the array between two points of the array. tempi = exp(linear_interpolation(alt, temp, size(alt), alti)) nuei = exp(linear_interpolation(alt, nue, size(alt), alti)) oxyi = exp(linear_interpolation(alt, oxy, size(alt), alti)) j = j + 1 !Integration integral = integral + tempi*nuei*oxyi*dz enddo end program main !Functions real function linear_interpolation(x, y, n, x0) implicit none integer :: n, i, k real :: x(n), y(n), x0, y0 k = 0 do i = 1, n-1 if ((x0 >= x(i)) .and. (x0 <= x(i+1))) then k = i ! k is the index where: x(k) <= x <= x(k+1) exit ! exit loop end if enddo if (k > 0) then ! compute the interpolated value for a point not in the array y0 = y(k) + (y(k+1)-y(k))/(x(k+1)-x(k))*(x0-x(k)) else write(*,*)'Error computing the interpolation !!!' write(*,*) 'x0 =',x0, ' is out of range <', x(1),',',x(n),'>' end if ! return value linear_interpolation = y0 end function linear_interpolation
I can provide a more detailed description of the exact errors, i was hoping that the error name would be enough since i have a few of each type.