FragmentPagerAdapter Swipe to show ListView 1/3 Screen Width
Solution 1
The following code achieves the desired effect:
In PageAdapter
:
@Override
public float getPageWidth(int position) {
if (position == 0) {
return(0.5f);
} else {
return (1.0f);
}
Solution 2
Reading your question one last time... make sure you also set up specific layouts for each size device. In your screenshots it looks like your trying to run this on a tablet. Are you getting the same results on a phone?
Setting up your Layout
Make sure your layout is simular to this and has the ViewPager:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="@color/body_background">
<include layout="@layout/pagerbar" />
<include layout="@layout/colorstrip" />
<android.support.v4.view.ViewPager
android:id="@+id/example_pager"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_weight="1" />
</LinearLayout>
Setting up your Activity
Setup your PagerAdapter in your "FragmentActivity" and make sure you implement "OnPageChangeListener". Then properly setup your PagerAdapter in your onCreate.
public class Activity extends FragmentActivity
implements ViewPager.OnPageChangeListener {
...
public void onCreate(Bundle savedInstanceState) {
PagerAdapter adapter = new PagerAdapter(getSupportFragmentManager());
pager = (ViewPager) findViewById(R.id.example_pager);
pager.setAdapter(adapter);
pager.setOnPageChangeListener(this);
pager.setCurrentItem(MyFragment.PAGE_LEFT);
...
}
/* setup your PagerAdapter which extends FragmentPagerAdapter */
static class PagerAdapter extends FragmentPagerAdapter {
public static final int NUM_PAGES = 2;
private MyFragment[] mFragments = new MyFragment[NUM_PAGES];
public PagerAdapter(FragmentManager fragmentManager) {
super(fragmentManager);
}
@Override
public int getCount() {
return NUM_PAGES;
}
@Override
public Fragment getItem(int position) {
if (mFragments[position] == null) {
/* this calls the newInstance from when you setup the ListFragment */
mFragments[position] = MyFragment.newInstance(position);
}
return mFragments[position];
}
}
...
Setting up your Fragment
When you setup your actual ListFragment (your listViews) you can create multiple instances with arguments like the following:
public static final int PAGE_LEFT = 0;
public static final int PAGE_RIGHT = 1;
static MyFragment newInstance(int num) {
MyFragment fragment = new MyFragment();
Bundle args = new Bundle();
args.putInt("num", num);
fragment.setArguments(args);
return fragment;
}
When you reload the listViews (how ever you decide to implement this) you can figure out which fragment instance you are on using the arguments like so:
mNum = getArguments() != null ? getArguments().getInt("num") : 1;
If you step through your code you will notice that it will step through each instance so the above code is only needed in your onCreate and a reload method may look like this:
private void reloadFromArguments() {
/* mNum is a variable which was created for each instance in the onCreate */
switch (mNum) {
case PAGE_LEFT:
/* maybe a query here would fit your needs? */
break;
case PAGE_RIGHT:
/* maybe a query here would fit your needs? */
break;
}
}
Few Sources that may help you out with examples that you could build from rather then starting from scratch:
More explanation and example from playground. http://blog.peterkuterna.net/2011/09/viewpager-meets-swipey-tabs.html which is references to: http://code.google.com/p/android-playground/
More info and some good linkage. http://www.pushing-pixels.org/2012/03/16/responsive-mobile-design-on-android-from-view-pager-to-action-bar-tabs.html
If you have more specific questions post and I can always Edit (update) my answer to address your questions. Good Luck! :)
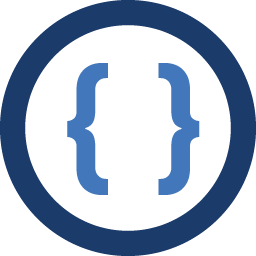
Admin
Updated on June 06, 2022Comments
-
Admin about 2 years
EDIT: See my answer below-->
I am wanting to have a view that when swiped to the right, the listView is shown. Very much similar to what is implemented in the new Google Play Store (Sample image below). I think its a ViewPager but I tried duplicating it without prevail. I was thinking it may just be that the 'listView Page' width attribute was set to a specific dp but that doesn't work. I also tried modifying pakerfeldt's viewFlow and cant figure out how Google does this
Am I on the right track? If someone has an idea how to duplicate this, I would greatly appreciate it. I think this may become a popular new way of showing a navigation view on tablets....? Code would be best of help. Thank you!!
Swipe right:
Finnished swipe; the layout shows the list and PART OF THE SECOND FRAGMENT (EXACTLY AS SHOWN) The list fragment does not fill the screen:
When the user swipes left, the main page is only shown and if the user swipes left again the viewPager continues to the next page.
-
Admin over 12 yearsThnx but that is what Iv'e been playing with too. I cant figure how to have fragment 0 (or the first page) not occupy the entire screen. Please see the Play Store app.
-
adneal over 12 yearsIf you're having layout problems, then adjust your layout. You have to inflate one for your
Fragment
. -
adneal over 12 yearsAh, wait. I think what you're talking about is the margin between
Fragments
. If that's the case, then usesetPageMargin()
withViewPager
to create space between eachFragment
. -
Neonfly over 11 yearsIt worked for me without problems. I got the same result as in the play-store. It only works for the most left fragment. In the onPageScroll events, you can steer the behaviour of the extended ViewPager. Use my
restrictLeftScroll()
function to get the same as in the play store. -
intrepidkarthi over 11 yearsGreat! There can't be a simple method than this :)